Getting Started
Basic Actions
Shortcutslang tries to be familiar to read and write. To write an action, put the action name with no spaces and a list of arguments in order.
ActionName "Action" "Arguments"
For example, the text action is named Text and has one field, the text inside it.
Text "This is my text"
Image
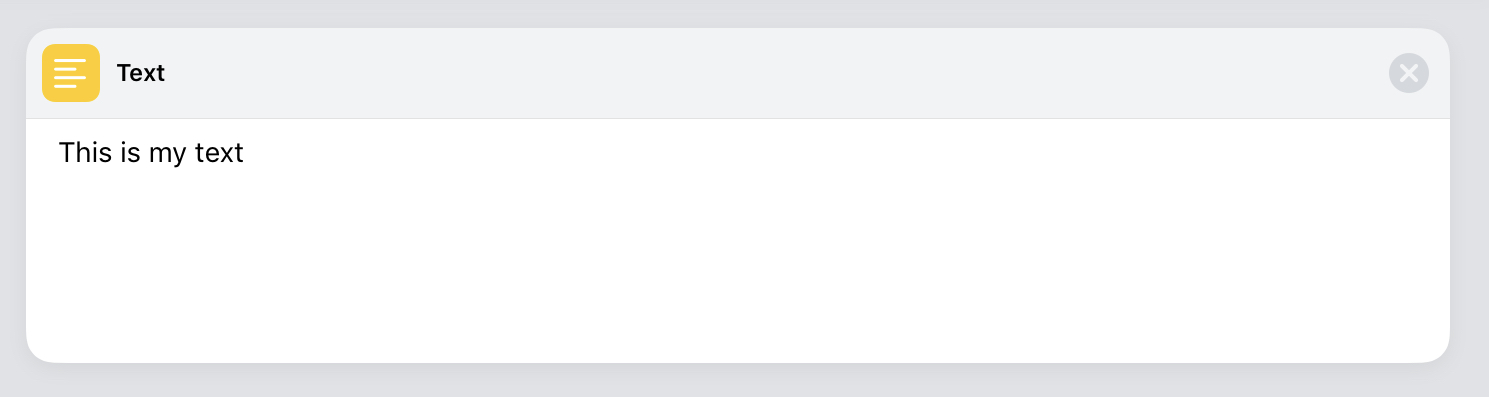
Actions shown | Text |
Try making shortcuts
You can convert ScPL to .shortcut on the tryit page. Copy the .shortcut file to your phone through airdrop or iCloud files and open it in Shortcuts.
Argument Labels
More complex actions have multiple fields
GetFile "iCloud Drive" true false
Image
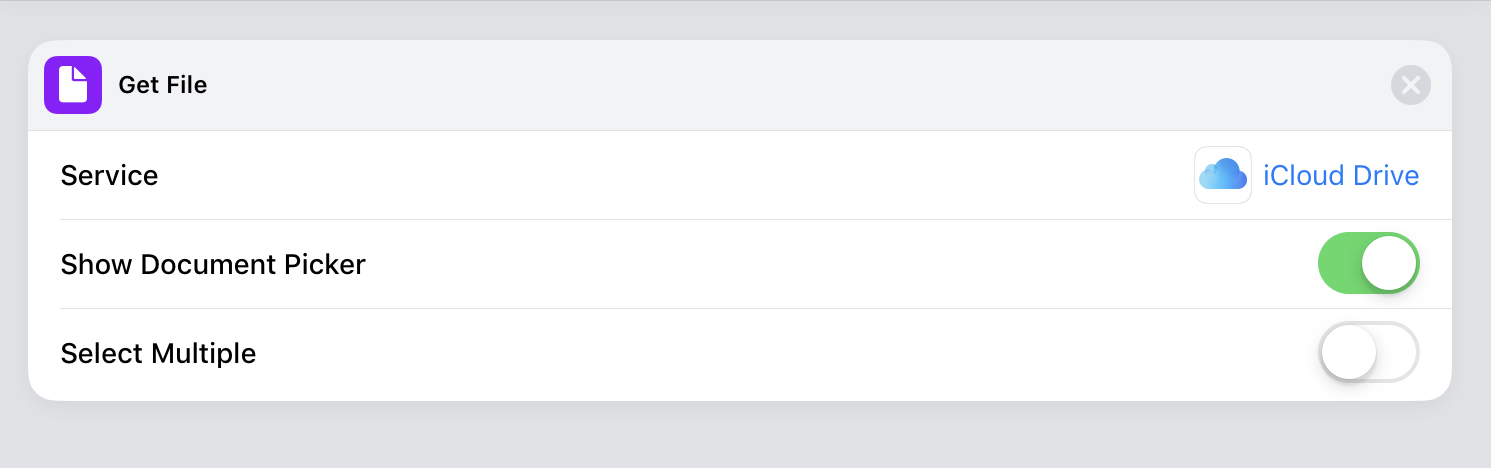
For these actions, you can put labels to know which field is which.
GetFile service="iCloud Drive" showdocumentpicker=true selectmultiple=false
Image
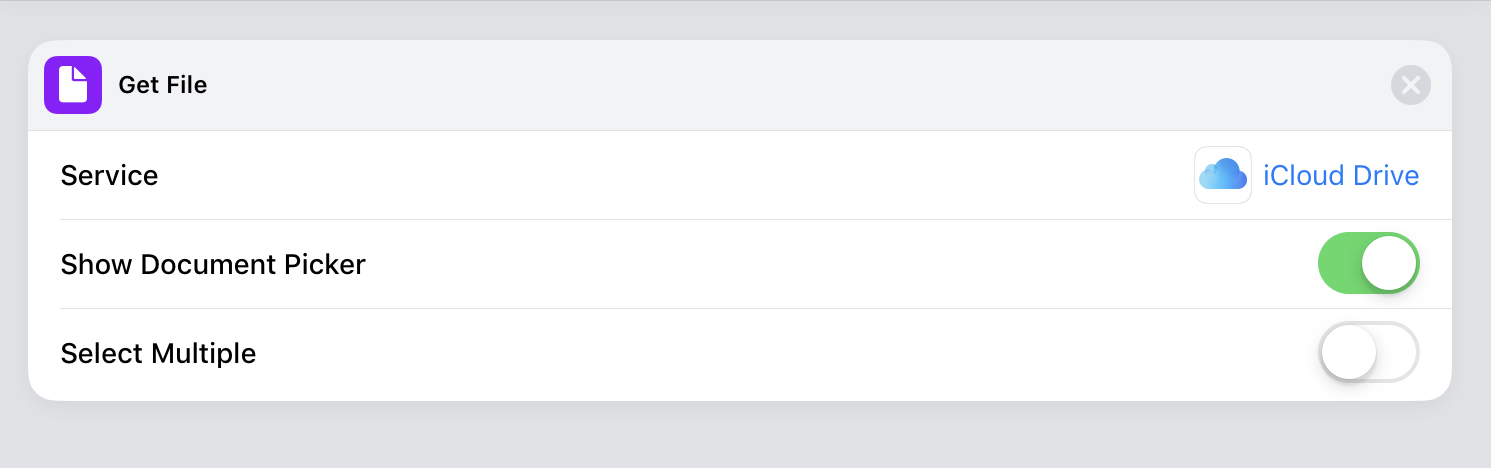
Actions shown | GetFile |
Block Actions
Other actions have blocks, like the if action and choose from menu action
Text "hello"
If "Equals" "hello"
ShowAlert title="Alert!" message="It equals hello"
Otherwise
ShowAlert title="Alert!" message="It does not equal hello"
End If
Image
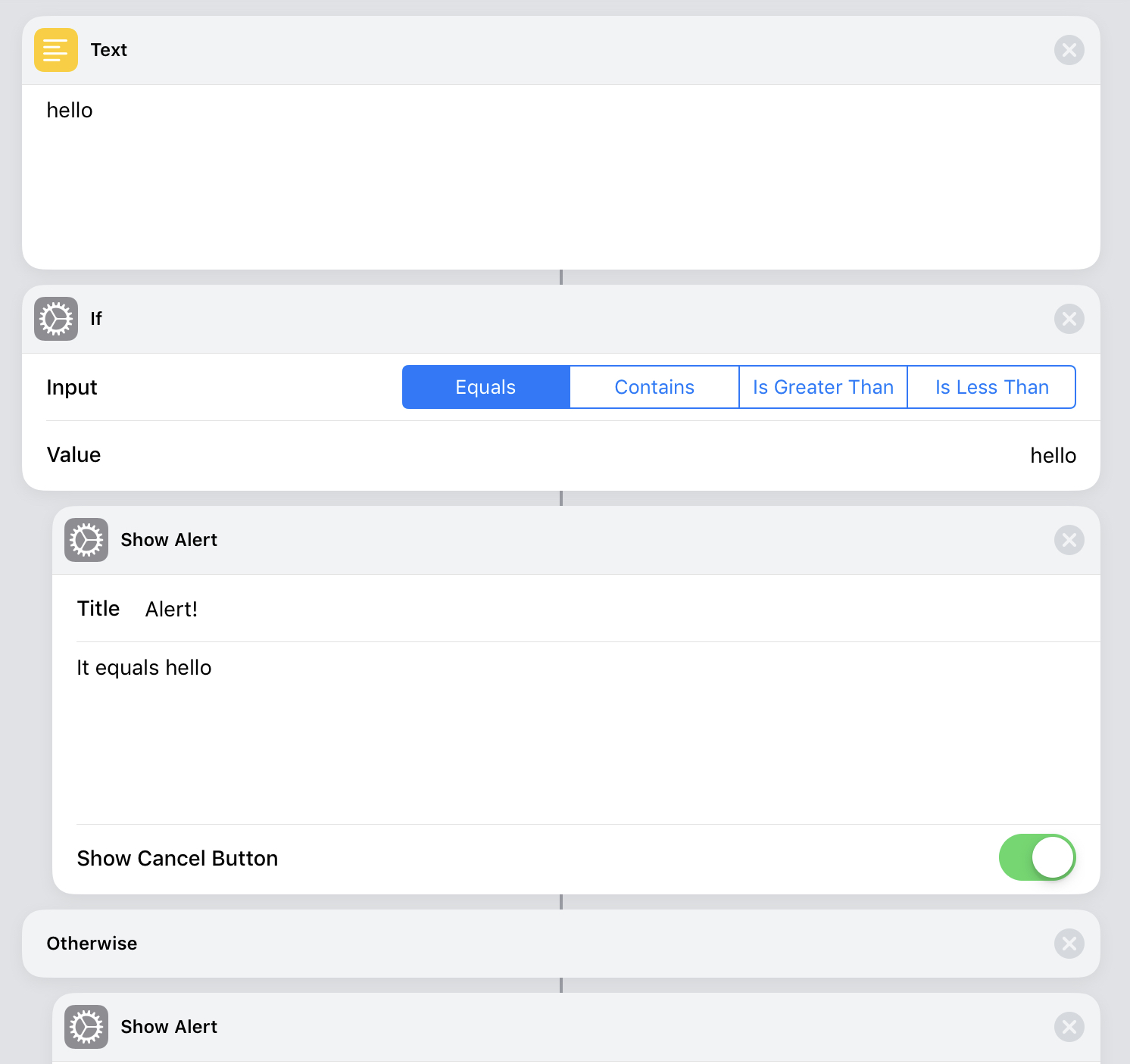
Actions shown | If | ShowAlert | Text |
Variables
Variables are marked with type:
varname. For example, a named variable called Input
would be v:Input
. There are 3 variable types, v
for named variable, mv
for magic variable, and s
for special variables. To use a variable with spaces in the name, surround the name with quotes like v:"Repeat Index"
Using Variables
Variables can be used directly as a field value to an action or they can be put within strings or barlists (more info below).
Text mv:MyMagicVariable
Text "Backslash parenthesis are used to put a variable inside text, like \(v:ThisVariable)."
Image
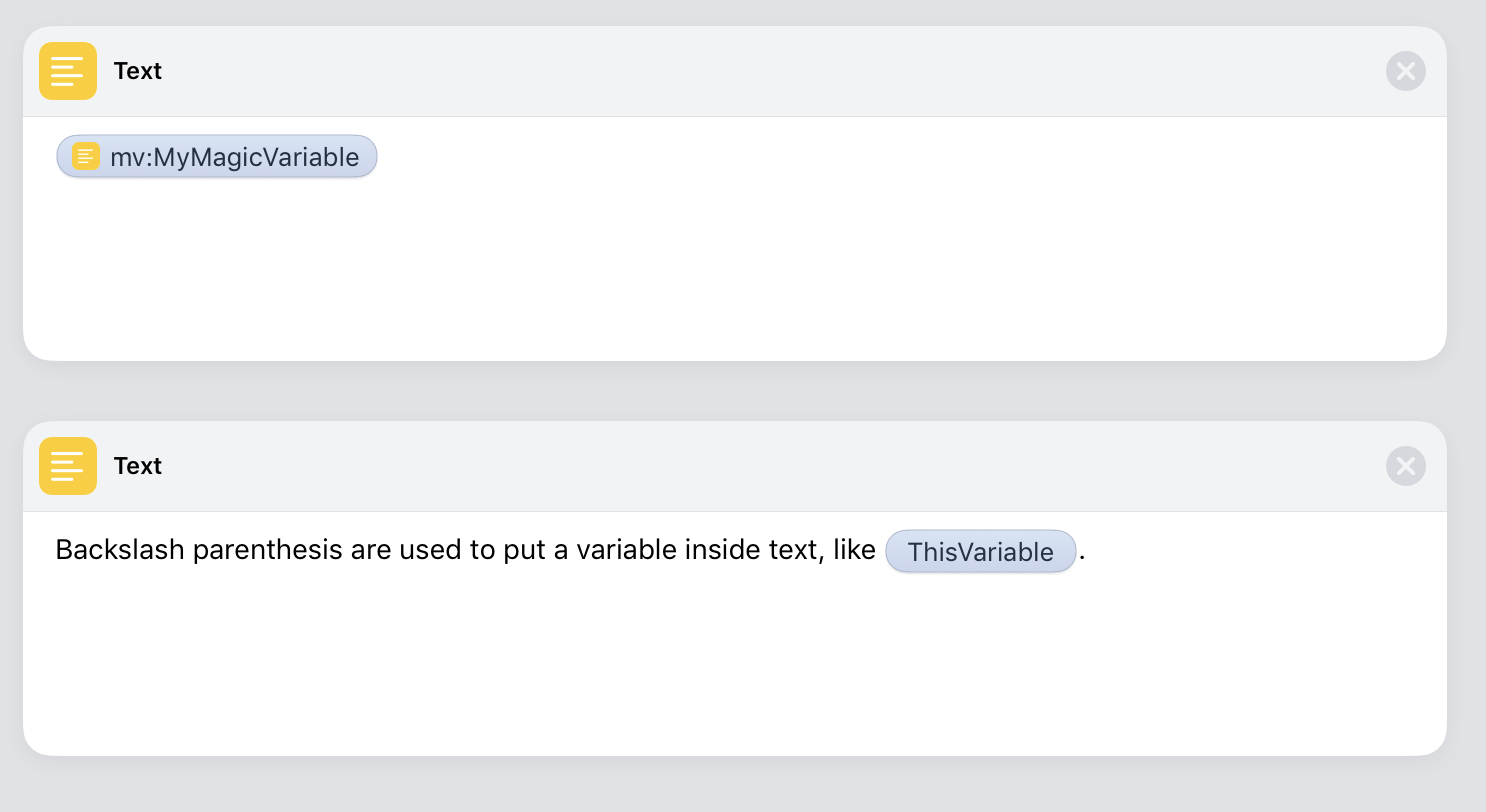
Text
| The same is used for \(v:Variables) in barlists.
Image
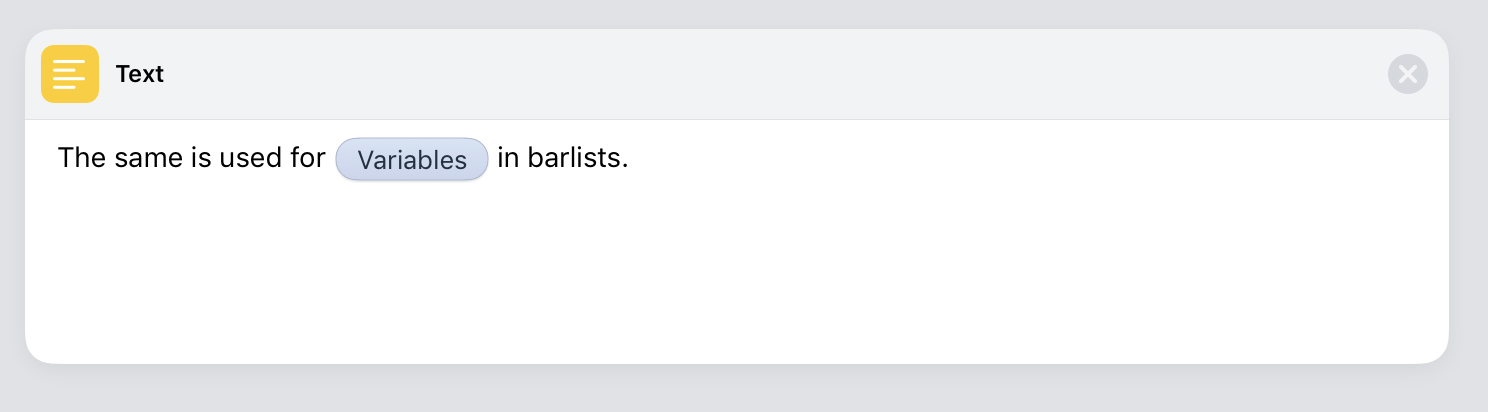
Actions shown | Text |
Setting Variables
Named variables are set just like how they are in shortcuts, using the SetVariable action. For example,
Text "My text"
SetVariable "MyVariable"
# now you can use it
ShowResult "My text is \(v:MyVariable)"
Image
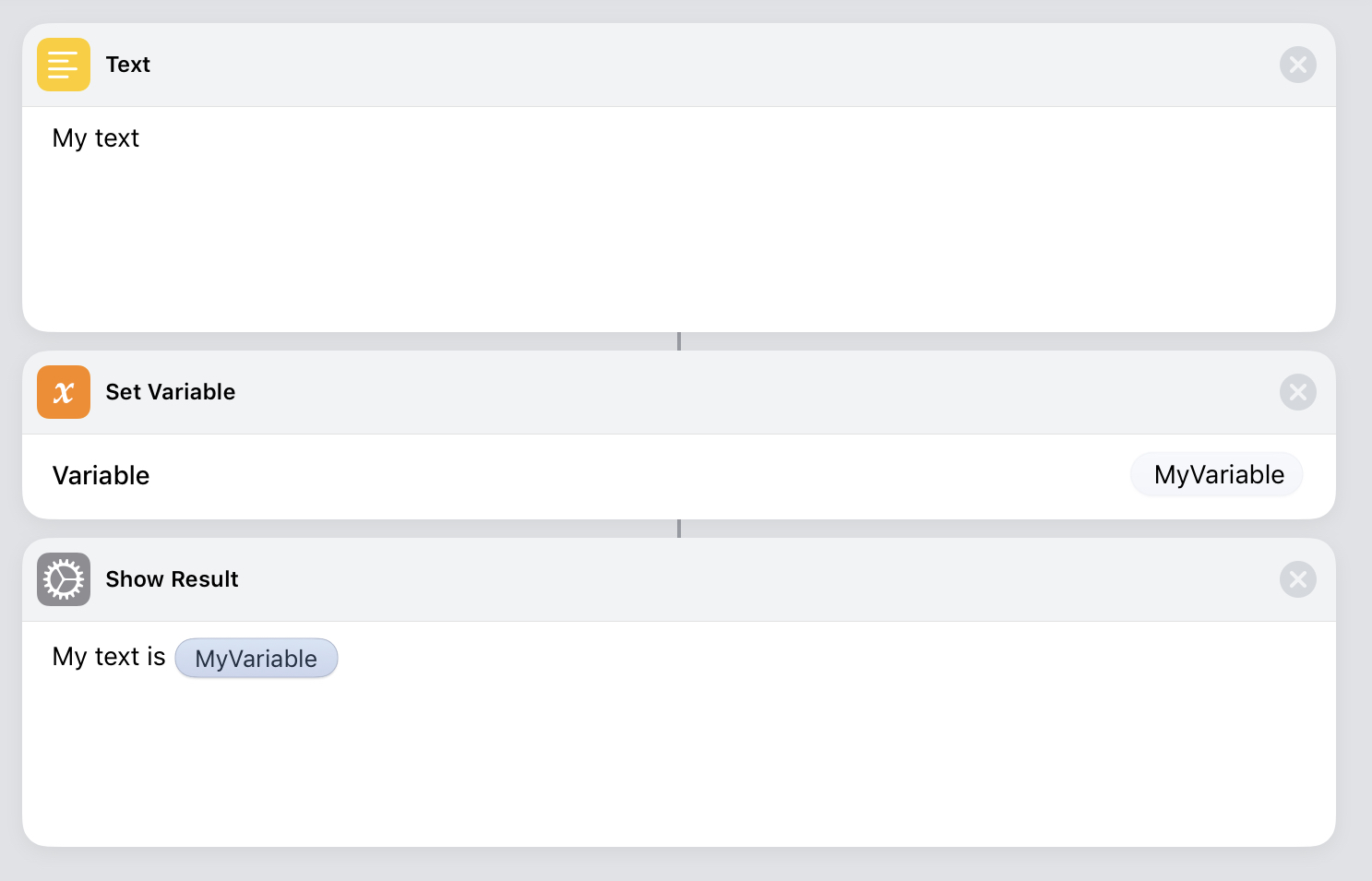
Magic variables are magical and don’t require an action to set. Instead you use the ->
arrow set syntax to say that the action is magical.
Text "My text" -> mv:Magic
# now you can use it
ShowResult "Magic! \(mv:Magic)"
Image
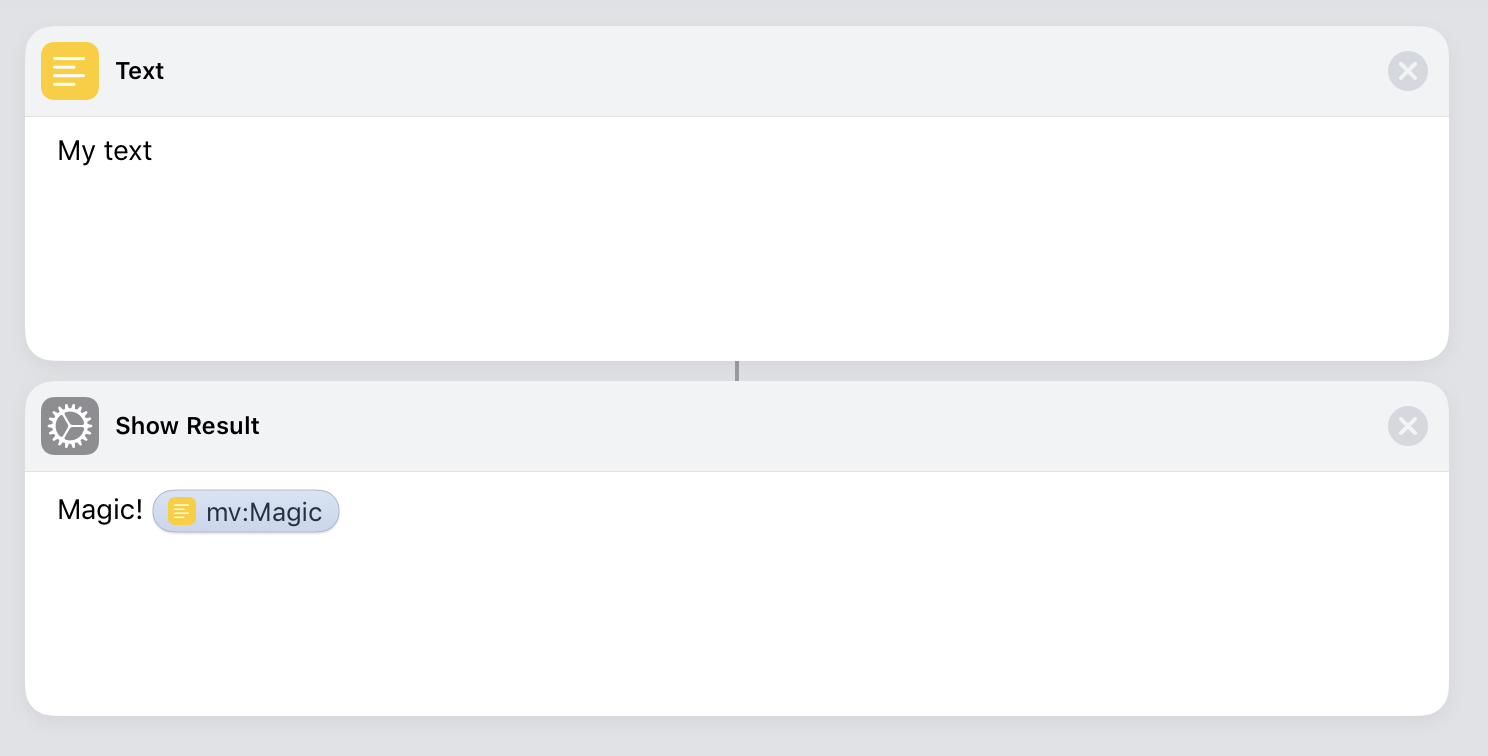
You cannot set special variables, they are special.
Actions shown | Text | ShowResult |
ScPL Comments
ScPL comments can be put in your code like shortcuts comments but they do not output any actions.
# this is a comment
// this is also a comment
-- this is also a comment
Image
This shortcut has no actionsField Types
Shortcuts has many different field types, for each field type there are many ways you can write them in shortcutslang
Text Field
Many actions have text fields of different sizes. The Text action has a multiline text field, and the Save file action has a single line text field to enter a destination path.
Text fields can be used with strings and multiline text fields can also be made with barlists
Text "This is a text field with a string"
Text
| This is a multiline text field using a barlist.
| This is the second line
| This is a variable: \(v:MyVariable)
Image
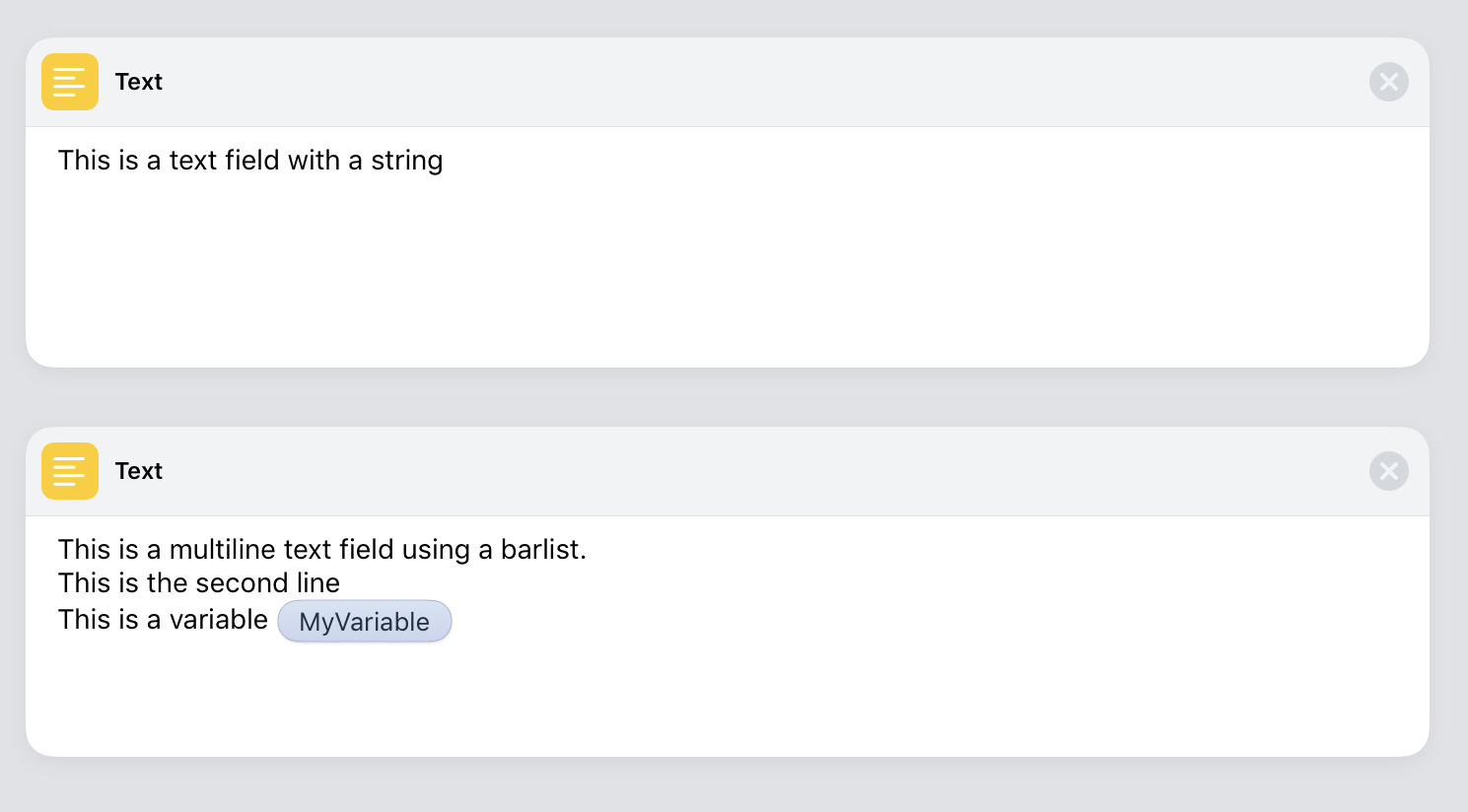
Some text fields do not allow variables, such as the Comment action.
Comment
| This text field does not allow variables
Image
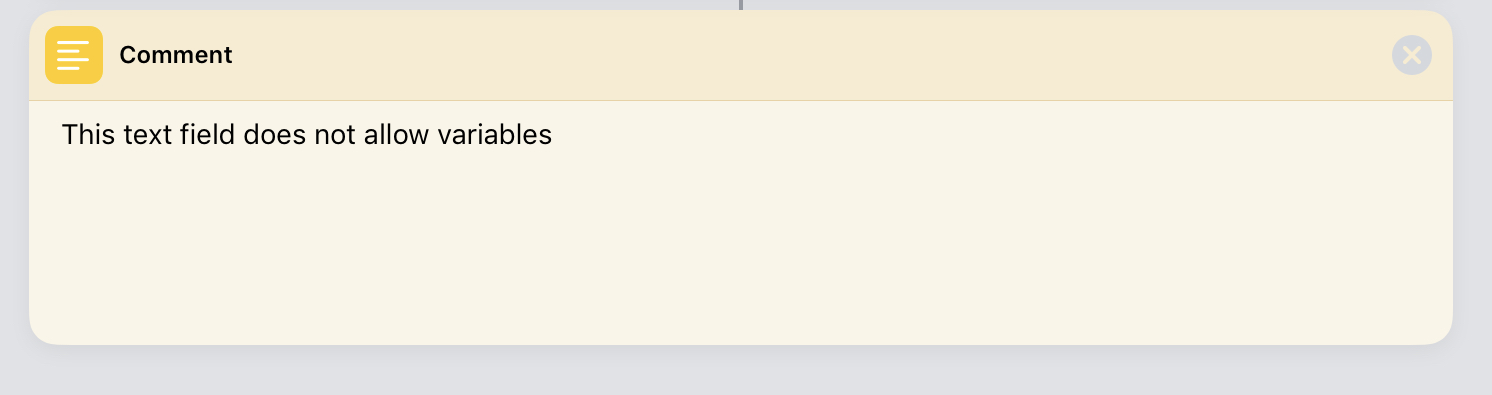
Comment
| \(v:Variable) # Not Allowed
Image
This shortcut fails to convertActions shown | Text | Comment |
Enum (Select) Field
An Enum Field has a list of options to choose from, such as the Condition field in if or the operator field in calculate.
Calculate "+" 1
Image
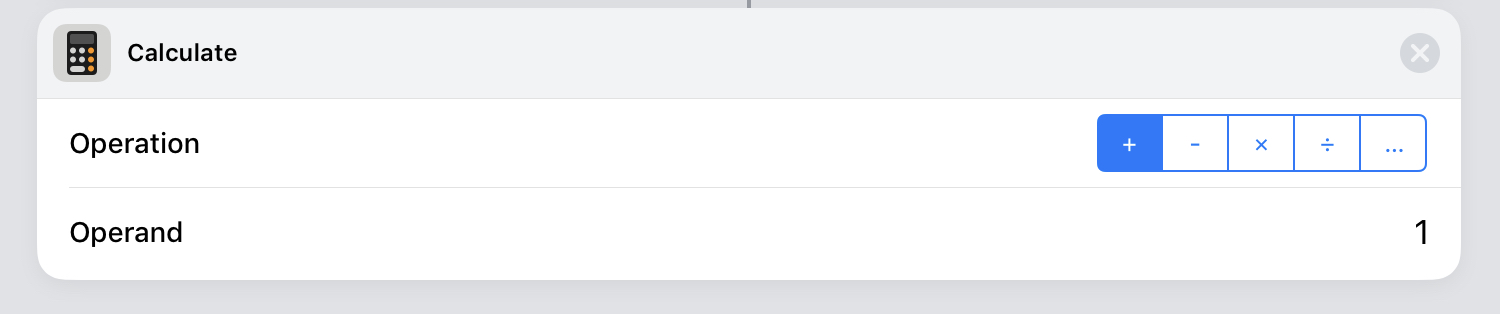
If you enter a value that is not allowed, shortcutslang will throw an error. Unlike action names, enum fields are case and space sensitive and must be exact.
Some enum fields allow variables, and some do not.
Calculate v:Operand 3
Image
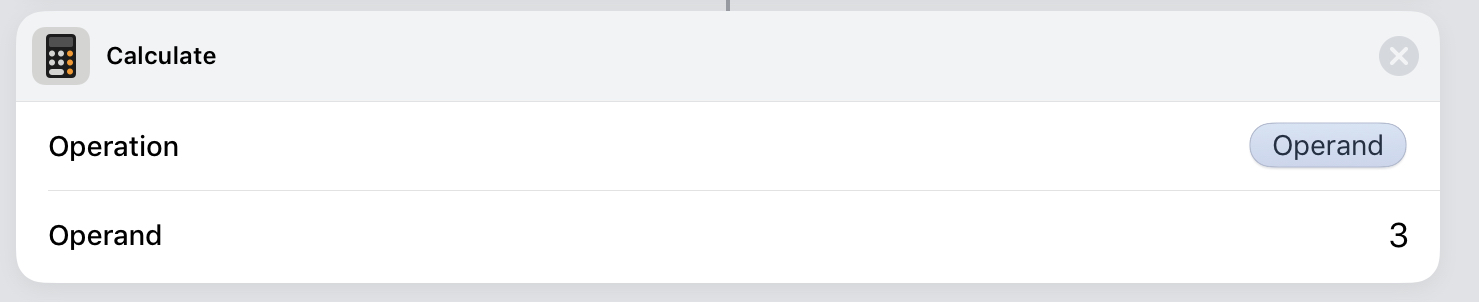
Actions shown | Calculate |
If an enum field does not allow variables, it will error when you try to use a variable.
Enum fields cannot have mixed text and variables, so "\(v:Not) Equals"
is never allowed.
Number Field
Number fields accept a number.
Number 16
Number 29.5
Number -924
Number .8
Number -.2
Image
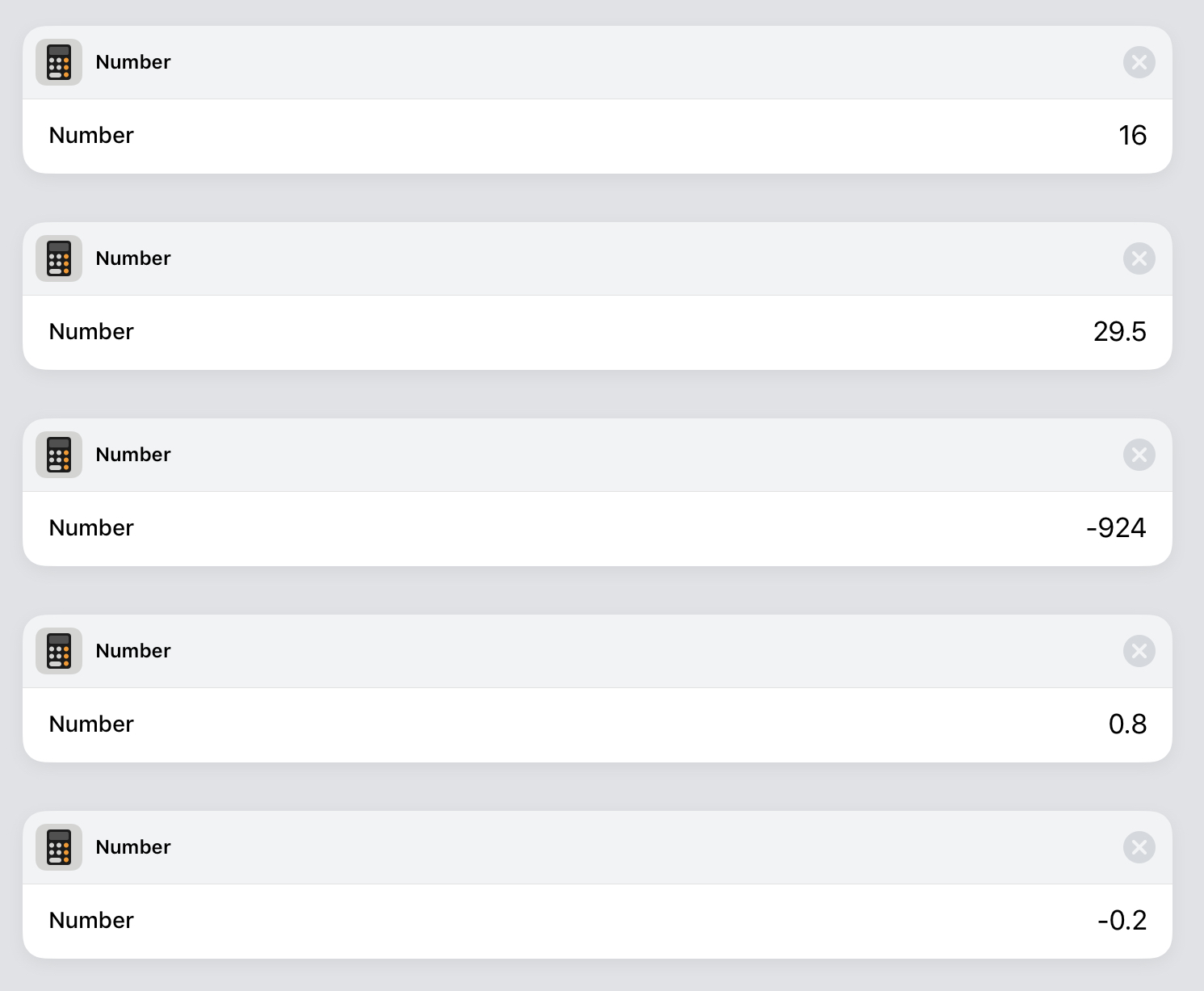
Similar to Enum fields, Number fields cannot have mixed text and variables.
Number v:MyVariable
Image

Number "-\(v:MyVariable)" # Not Allowed
Image
This shortcut fails to convertActions shown | Number |
Stepper Number Fields
Stepper number fields are like number fields but can only have positive integer numbers (no decimals)
Repeat 5
ShowResult "Hi"
End Repeat
Image
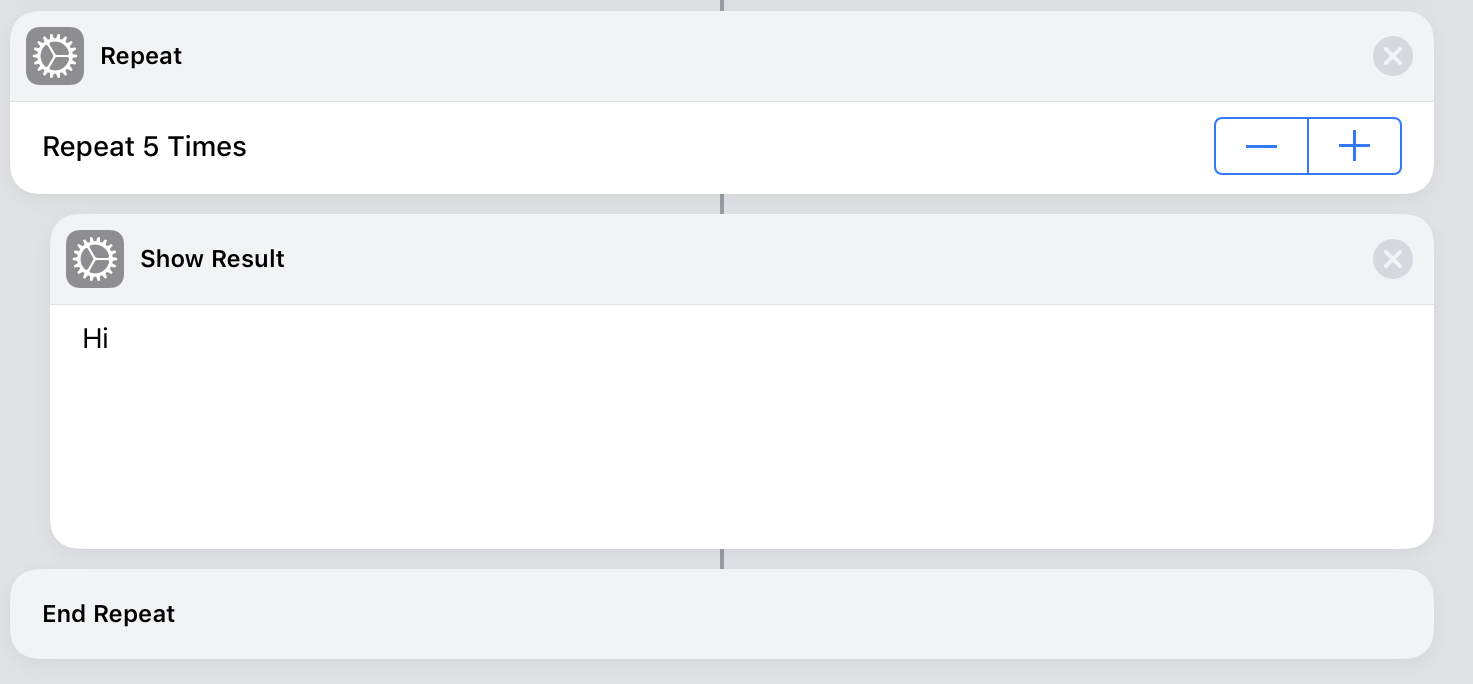
Repeat 5.5 # Not allowed
ShowResult "Hi"
End Repeat
Image
This shortcut fails to convertActions shown | Repeat |
Slider Number Fields
Slider number fields are like number fields but can only have numbers from 0 to 1
SetBrightness 0.5
Image

SetBrightness 1.1 # Not Allowed
Image
This shortcut fails to convertActions shown | SetBrightness |
Variable Picker Fields
Variable picker fields can have a variable.
GetVariable v:MyVariable
Image

GetVariable "MyVariable" # Not Allowed
Image
This shortcut fails to convertActions shown | getvariable |
Switch (or Expanding or Boolean) Fields
Switch fields can have a true or false value. True is on and false is off.
SetWifi true
SetAirplaneMode false
Image
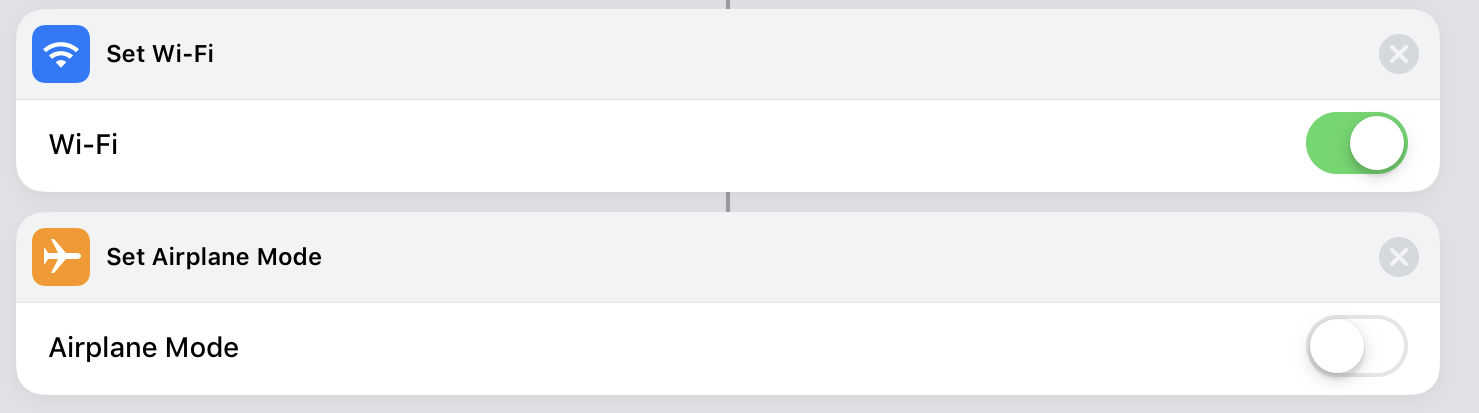
SetWifi yes # Not Allowed, must be true or false
SetAirplaneMode no # Not Allowed, must be true or false
Image
This shortcut fails to convertSome switch fields allow variables
SetWifi v:Wifi
Image

Actions shown | SetWifi | List |
Dictionary Field
Dictionary fields are written like JSON
Dictionary{
"key": "value",
"variable": v:myVariable,
"list": ["item 1", "item 2"],
"dictionary": {"key": "value"}
}
Image
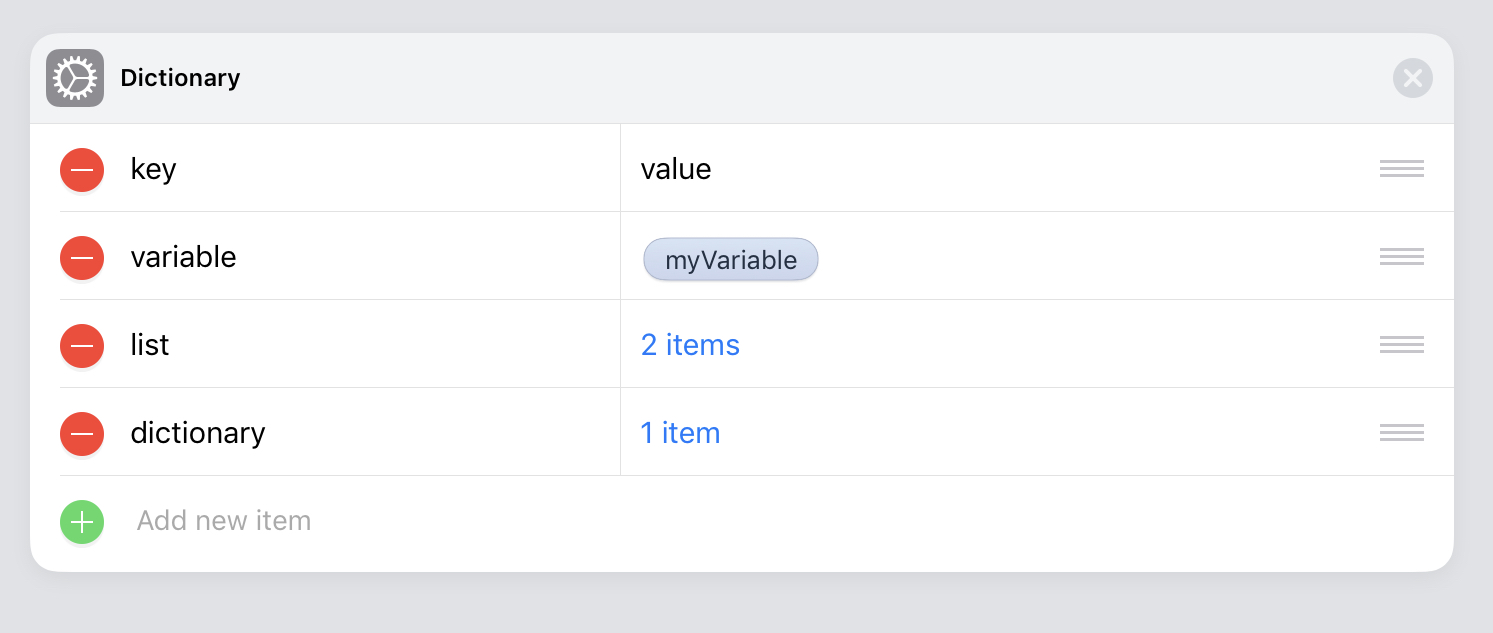
Quotes and commas are not required and =
can be used instead of :
if wanted
Dictionary{
key = value
variable = v:myVariable
list = ["item 1", "item 2"]
dictionary = {key = value}
}
Image
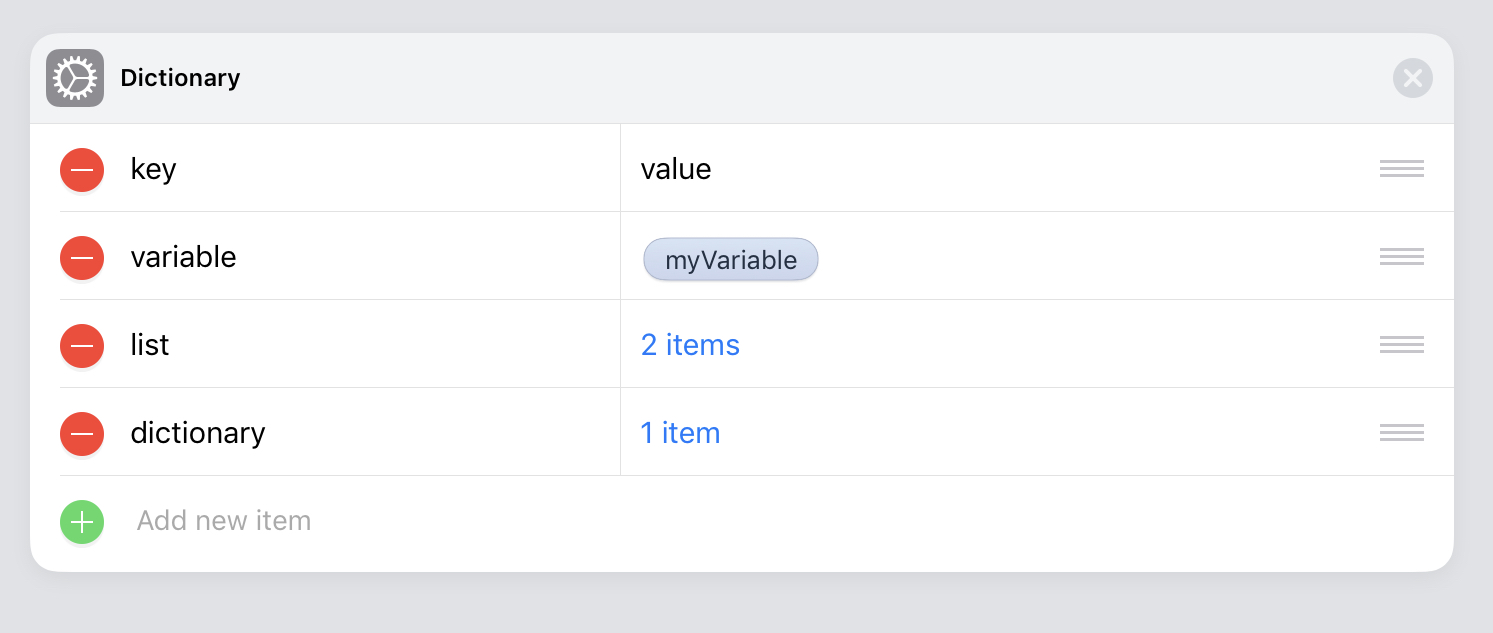
Actions shown | Dictionary |
List Field
Lists can be made using json-like []
syntax.
ChooseFromMenu "Pick an item" ["item 1", "item 2"]
case 1
case 2
end menu
Image
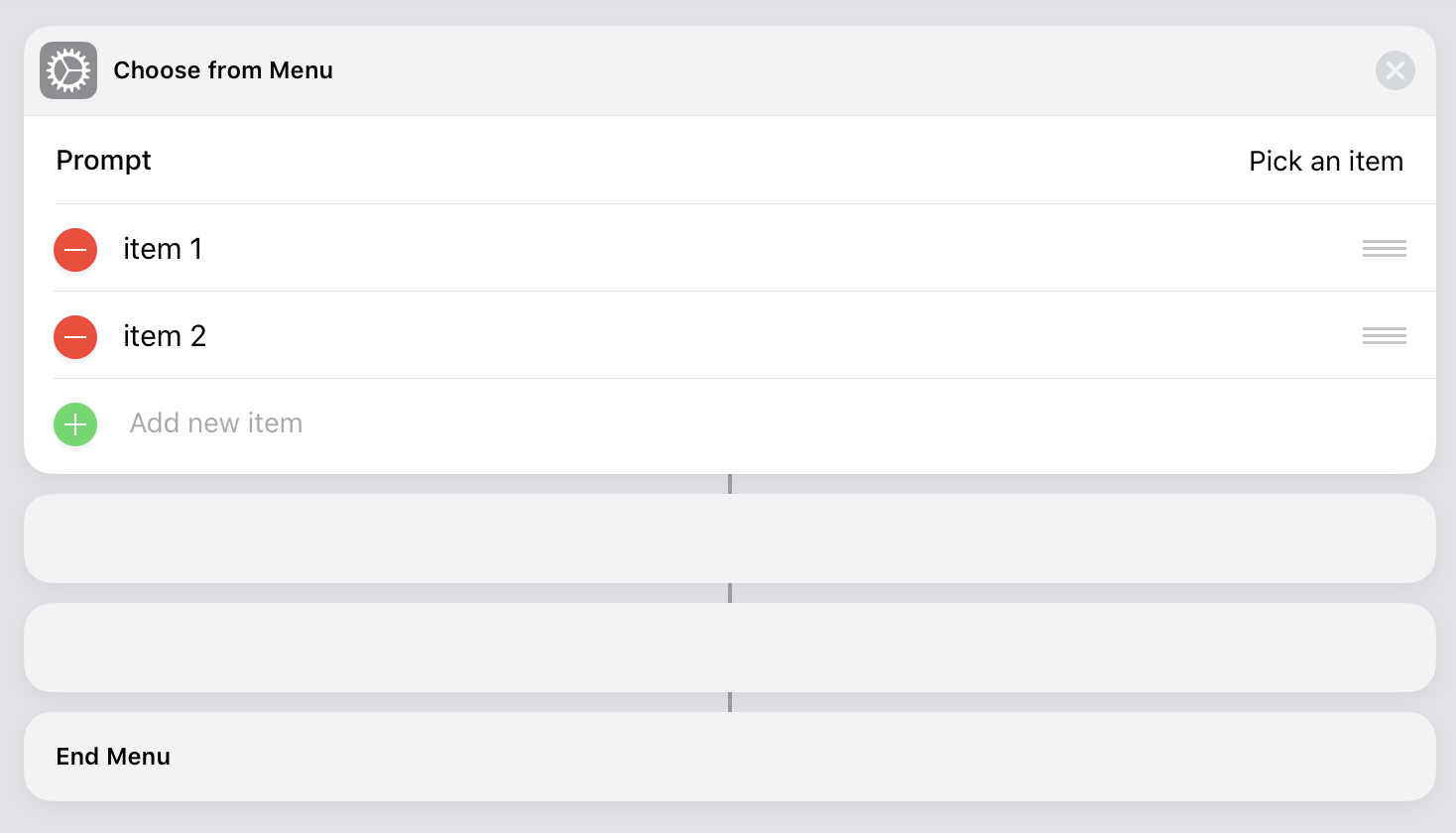
Similar to dictionaries, lists do not require commas and can span over multiple lines
ChooseFromMenu "Pick an item" [
"item 1"
"item 2"
]
case First
case Second
end menu
Image
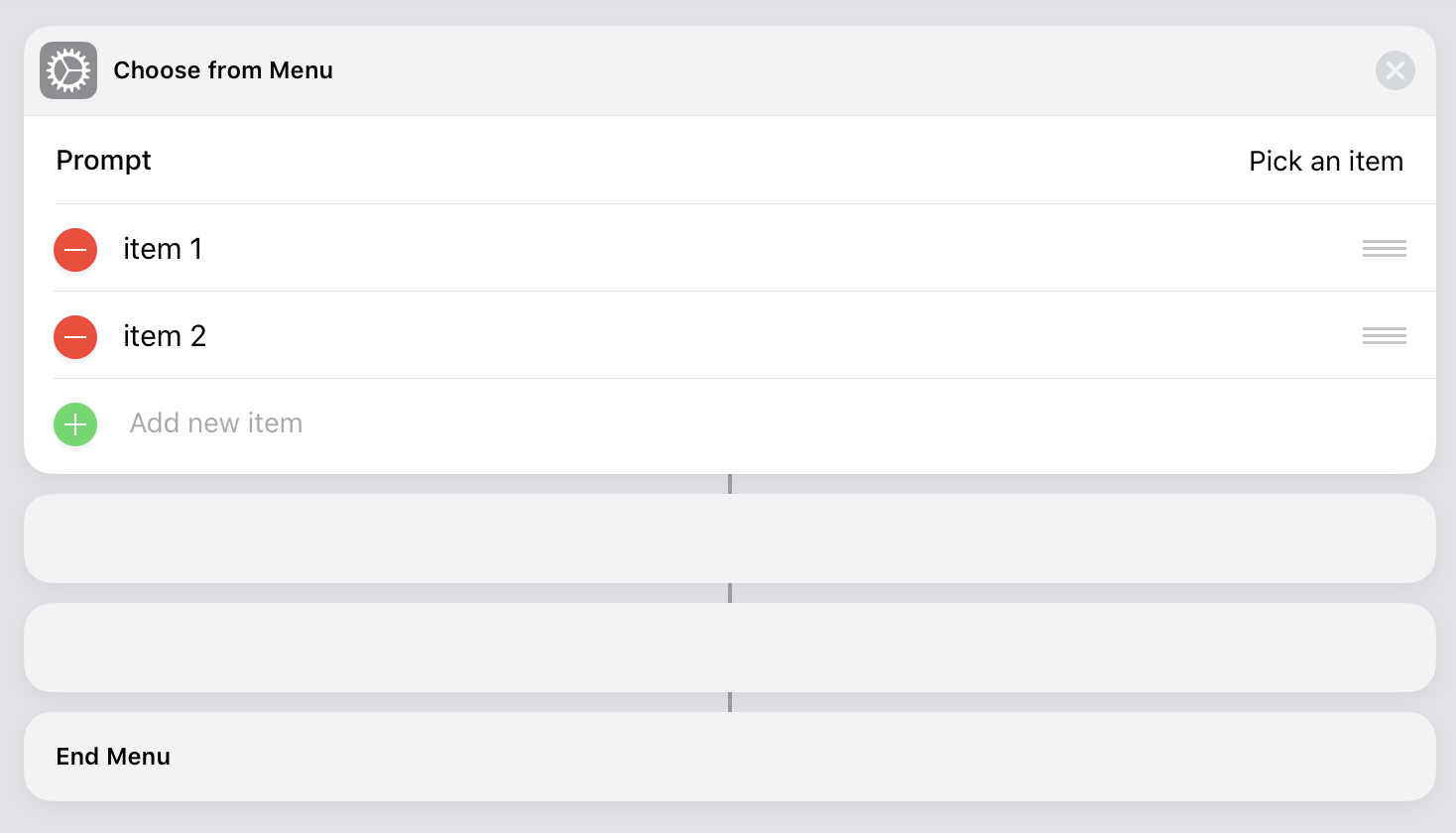
Lists can also be made using barlists.
List
| My First Item
| My Second Item
Image
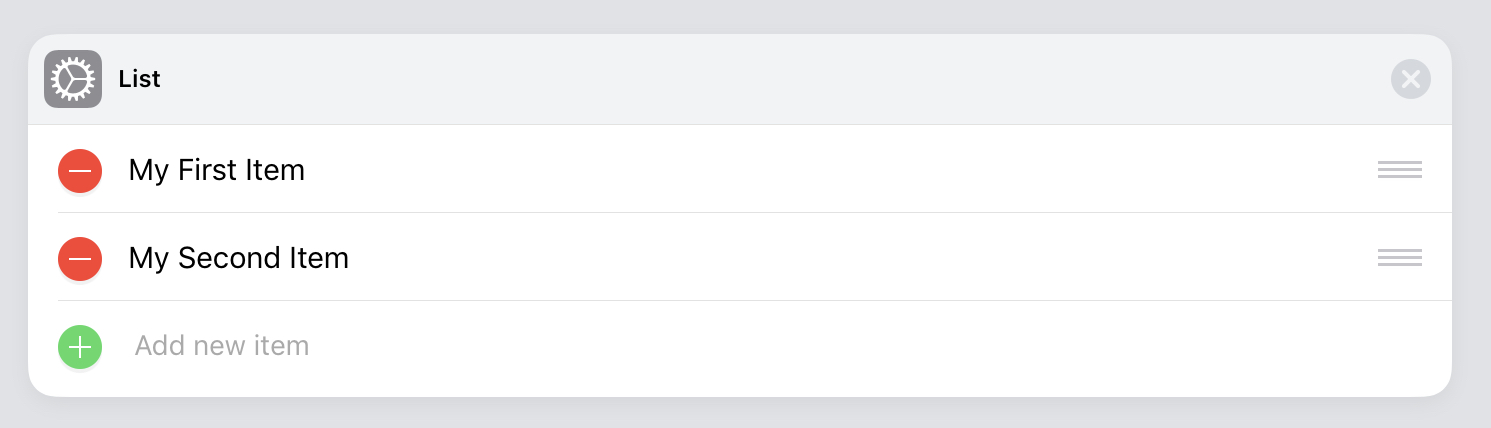
Actions shown | ChooseFromMenu | List |
Variable Field
(Different from Variable Picker Fields)
Variable fields is the field used in SetVariable to enter a variable name. They can be made using strings or v: variables.
SetVariable "MyNamedVariable"
SetVariable v:MyNamedVariable
Image
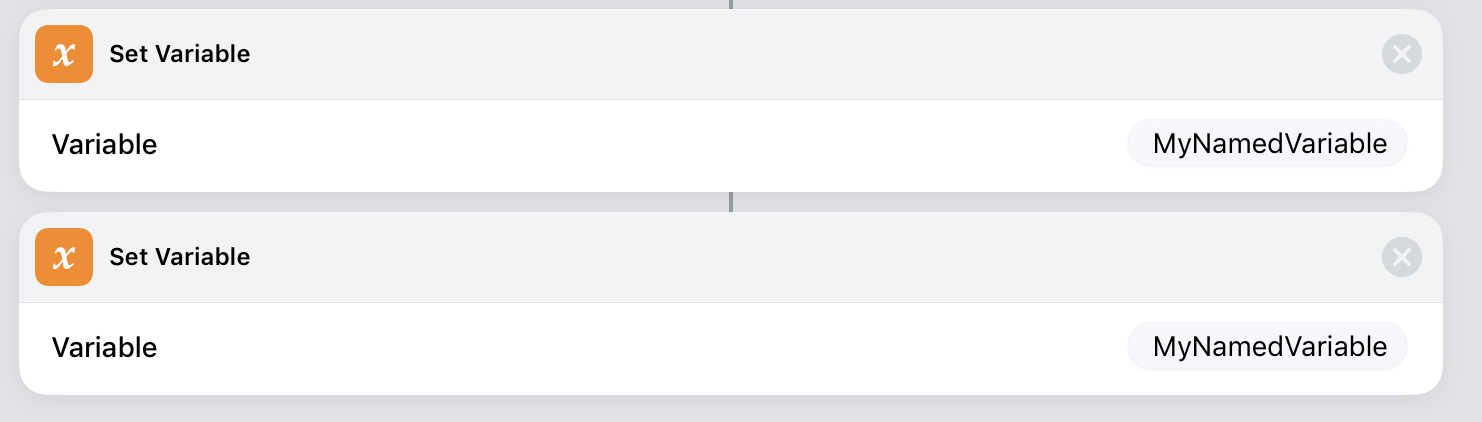
Filter Field
Filter fields
FilterFiles :filter{name is "filename"}
Actions shown | Filter Files |
Other Fields
There are other fields, most are very similar to similar fields like the Storage Service Picker is very similar to the Enum field.
Advanced Features
Shortcutslang has some more advanced features to make working on large shortcuts easier
Actions inside actions
You can put an action inside of a text field or any other field that accepts variable
Text "My number is \(Number -2.5)"
Image
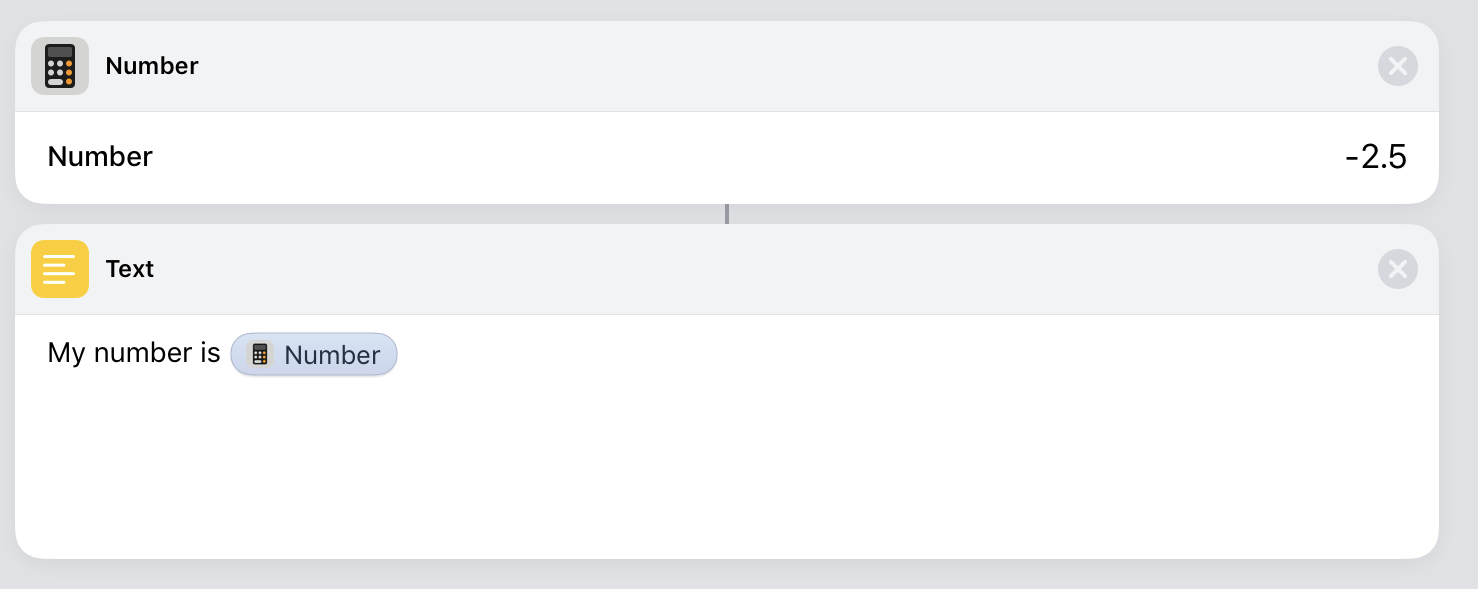
This code is equivalent to
Number -2.5 -> mv:InsideParenthesis
Text "My number is \(mv:InsideParenthesis)"
Image
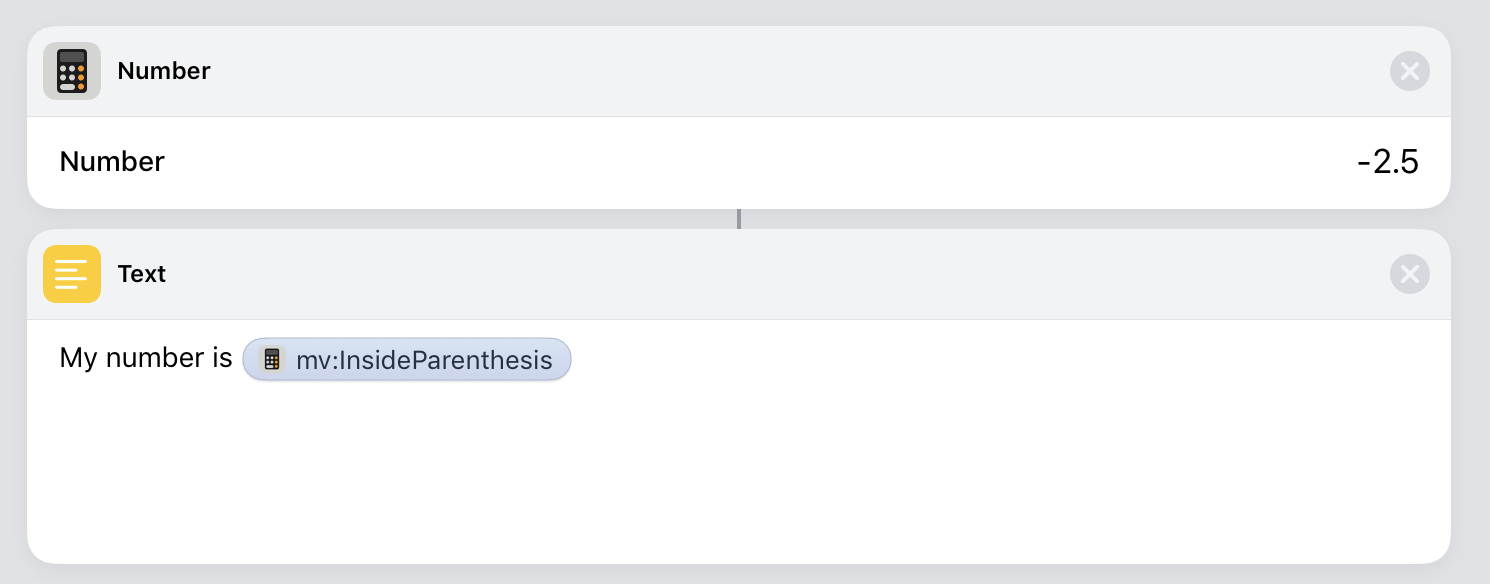
None of these magic variables actually get a name, they just directly reference the action.
You can even use actions inside other actions this without disrupting the action input
Text "Hello"
If Equals (Text "Goodbye")
ShowResult "If the action input was disrupted, this would run"
Otherwise
QuickLook
End If
Image
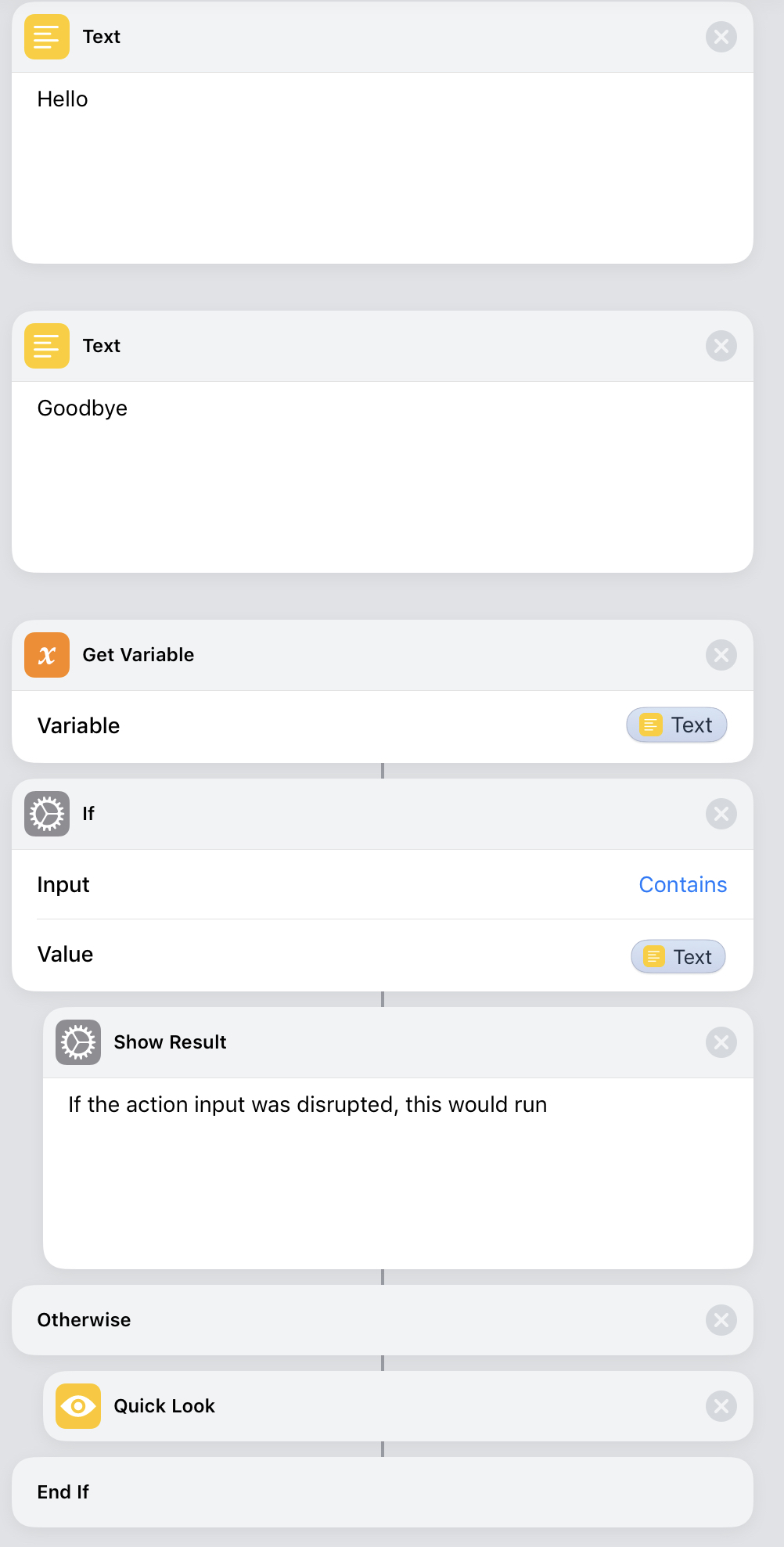
is equivalent to writing out
Text "Hello" -> mv:OriginalInput
Text "Goodbye" -> mv:ArgumentValue
GetVariable mv:OriginalInput
If Equals mv:ArgumentValue
ShowResult "If the action input was disrupted, this would run"
Otherwise
QuickLook # Shows "Hello"
End If
Image
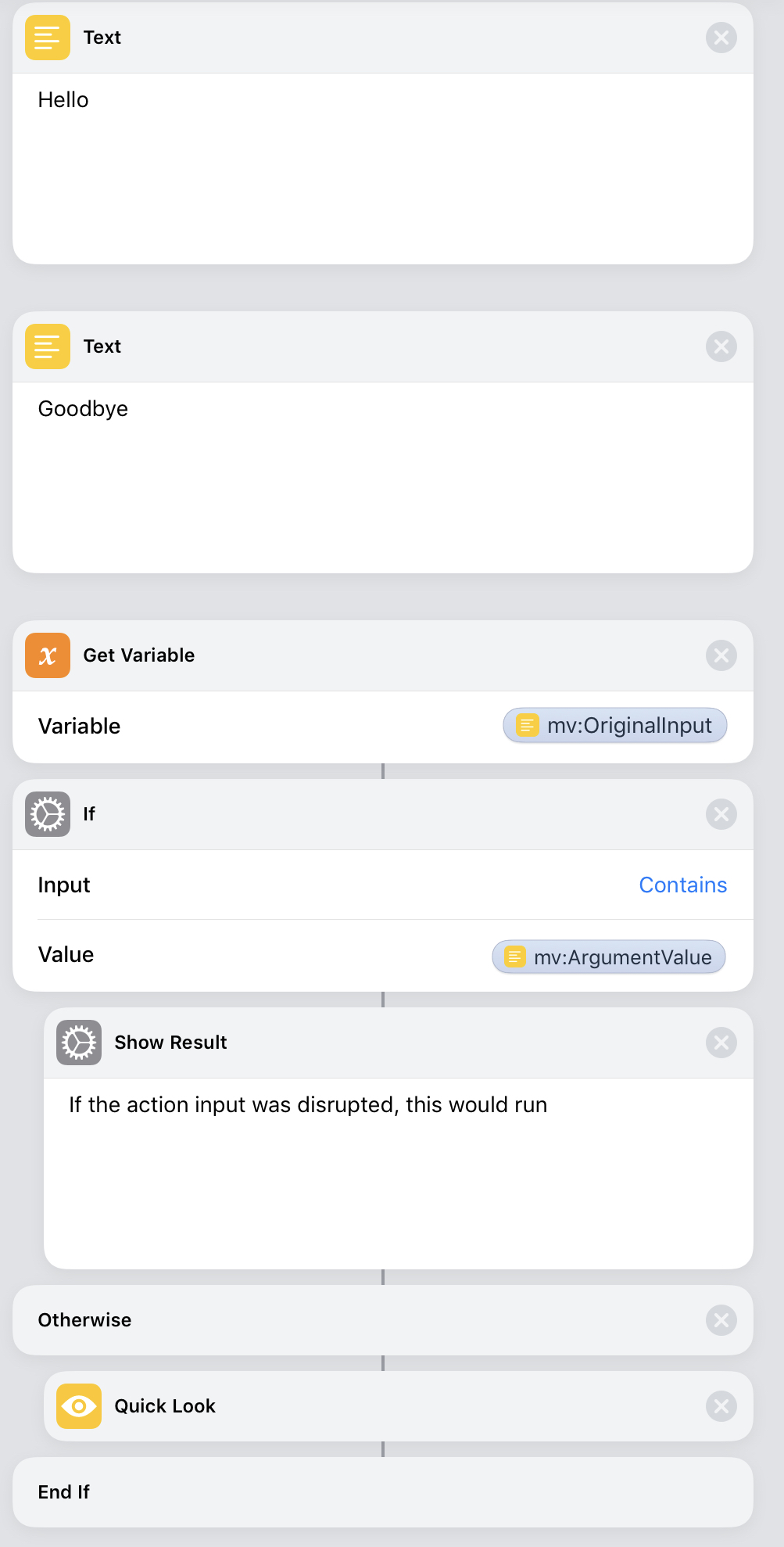
Actions shown | Text | GetVariable | If | QuickLook | Number |
InputArg
Sometimes you want to disrupt the input, you can use an InputArg
Text "Some text"
QuickLook ^(Text "Different text")
Image
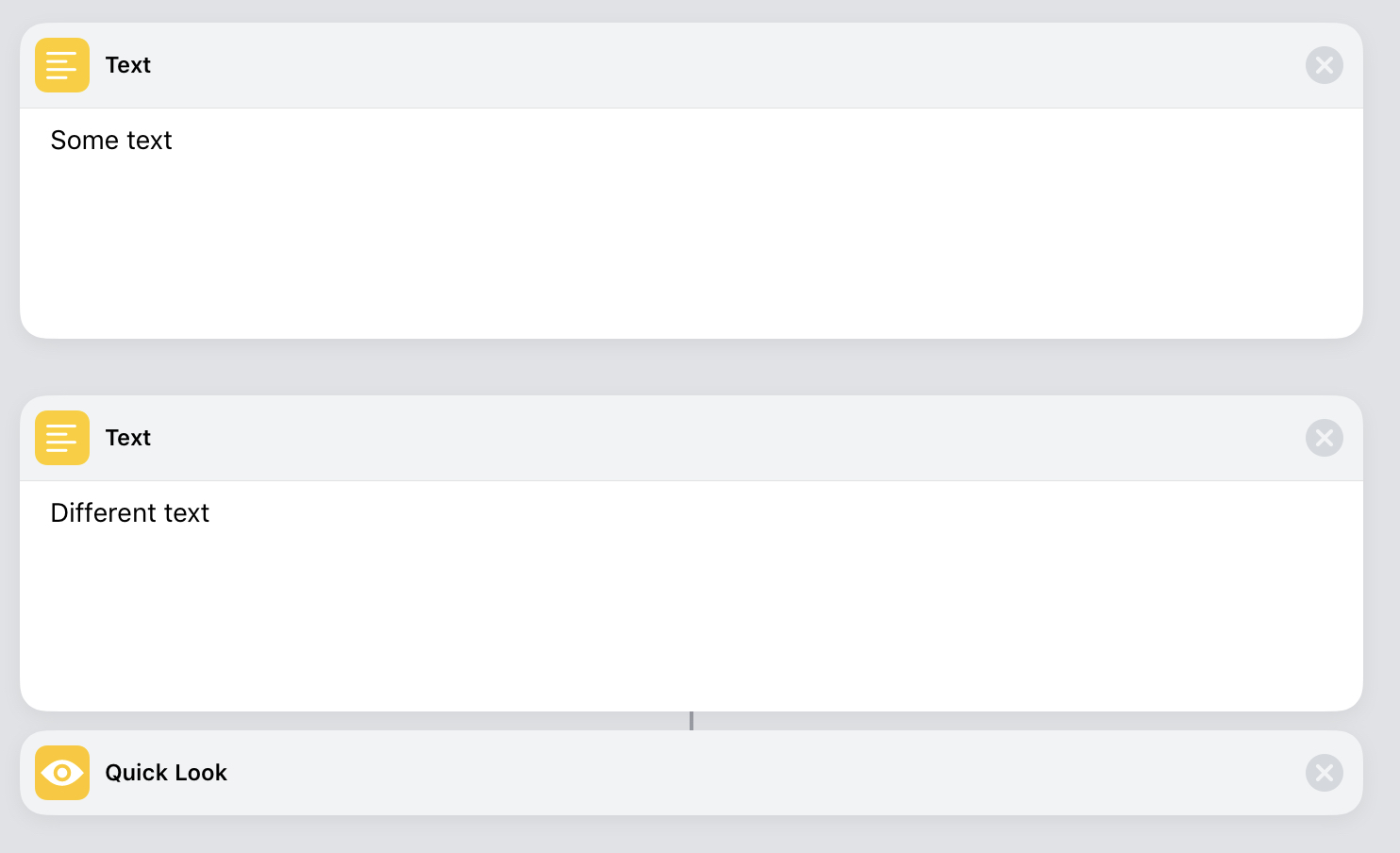
is the same as
Text "Some text"
Text "Different text"
QuickLook
Image
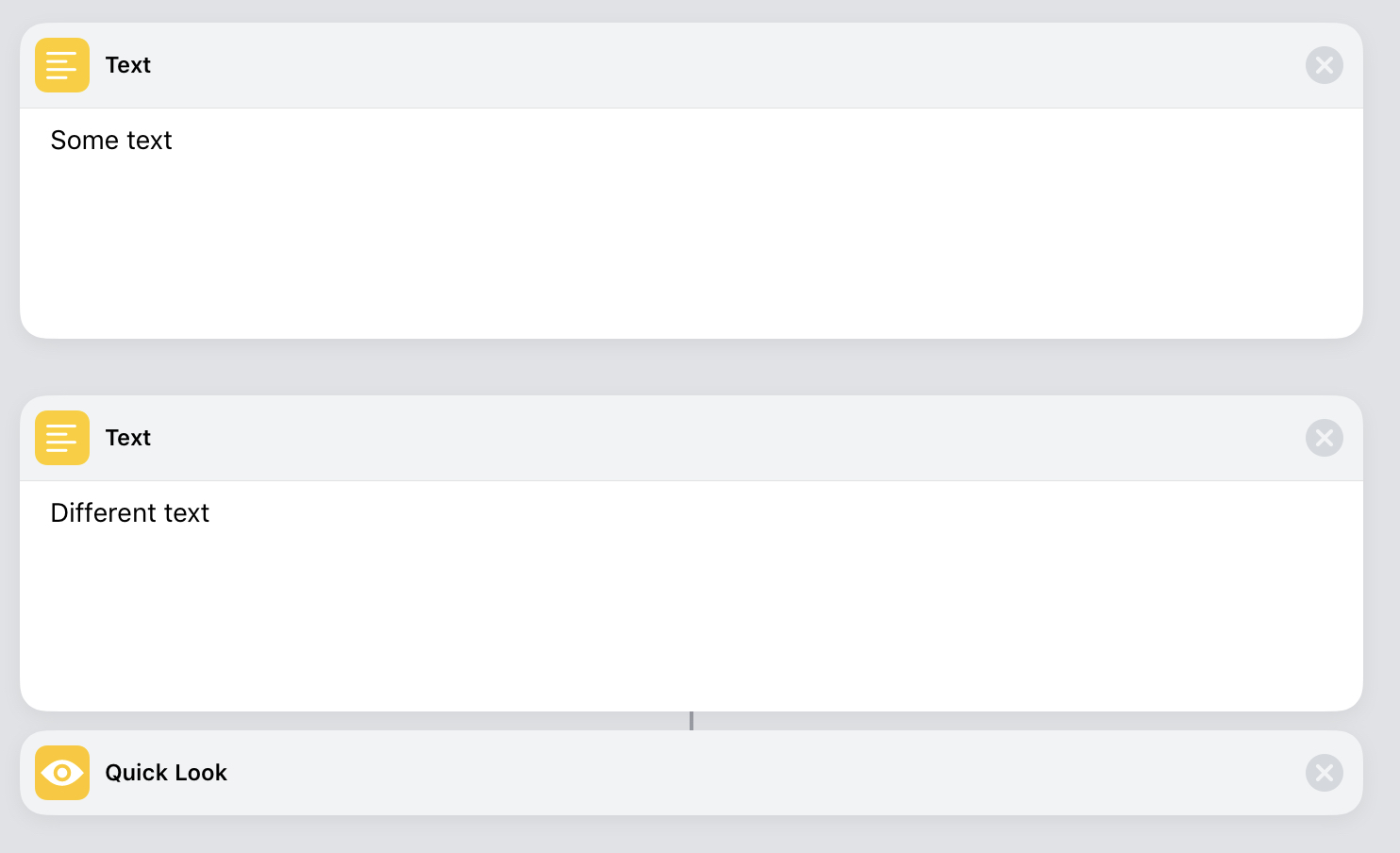
Actions shown | Text | QuickLook |
Variable Aggrandizements
When you tap a variable in Shortcuts, a menu pops up containing different actions. Those are called Aggrandizements.
GetVariable v:MyVariable{as:Dictionary,key:myKey}
GetVariable v:MyContact{as:Contact,get:Notes}
Image
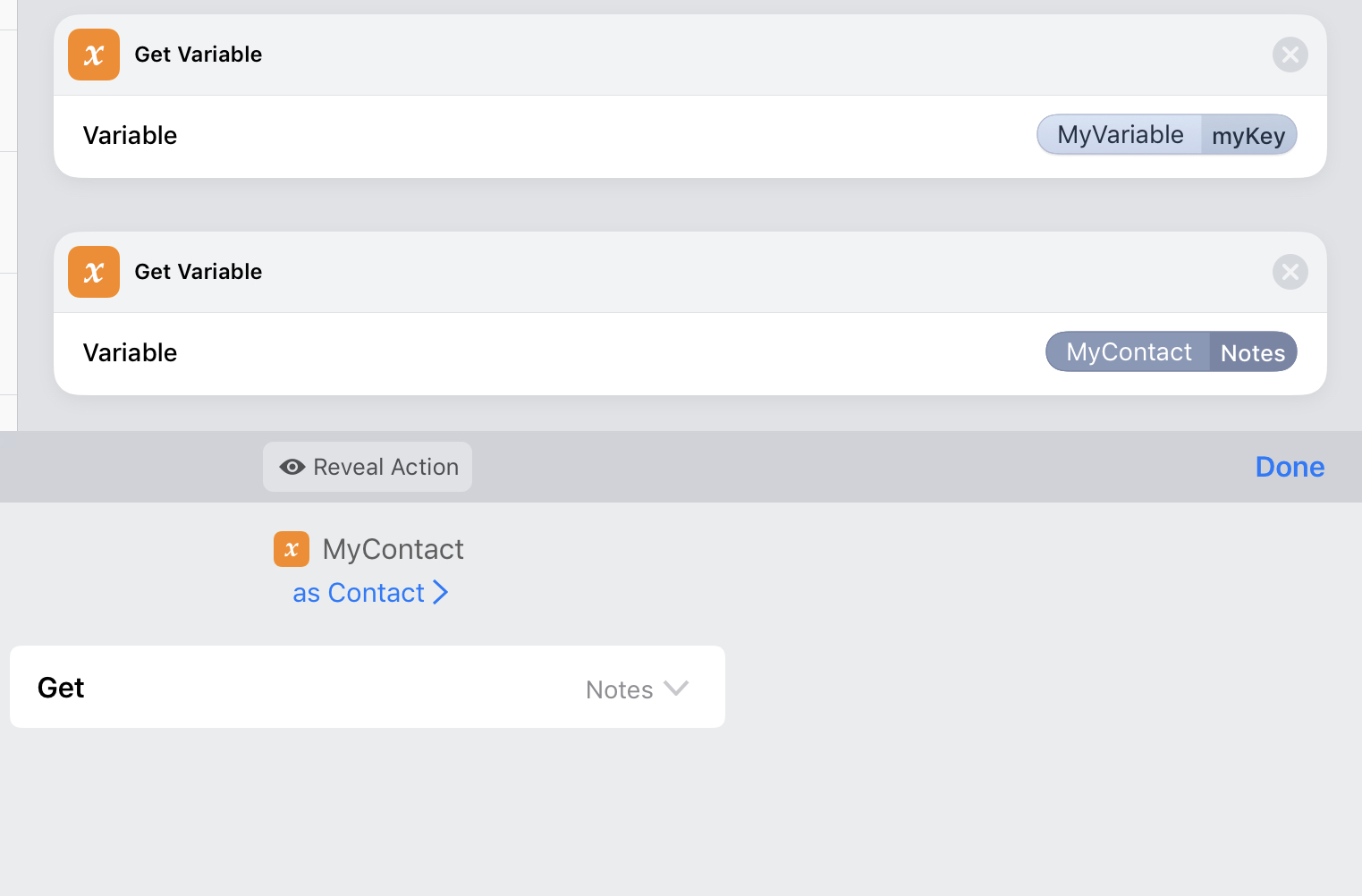
Currently, 3 aggrandizements are supported:
- as/coerce
- key/forKey
- get/getProperty (partial)
The key: aggrandizement is used very often in conjunction with the as: Dictionary aggrandizement. Instead of writing that out every time, there is a shortcut for it
GetVariable v:MyDictionary:myKey
Image
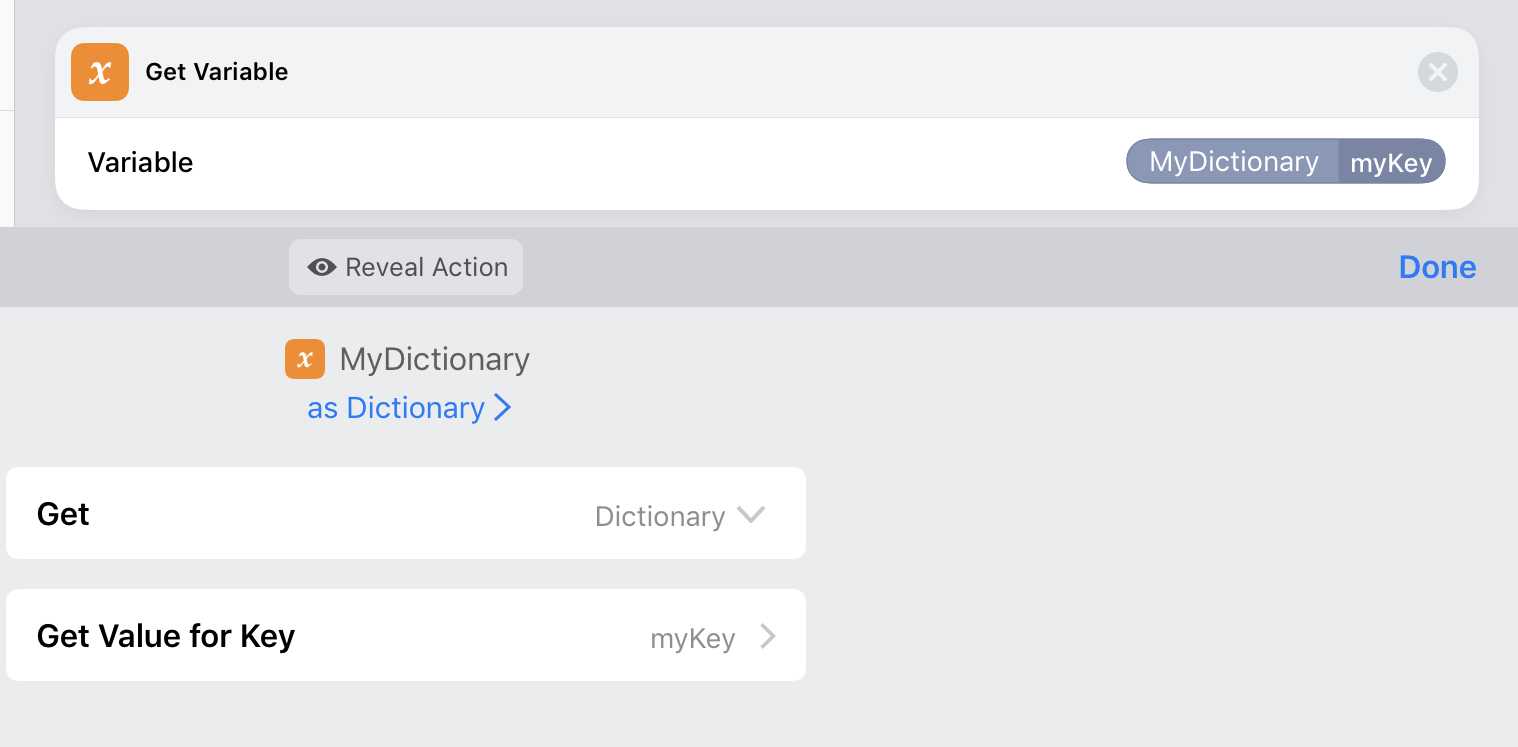
is the same as
GetVariable v:MyDictionary{as:Dictionary,key:myKey}
Image
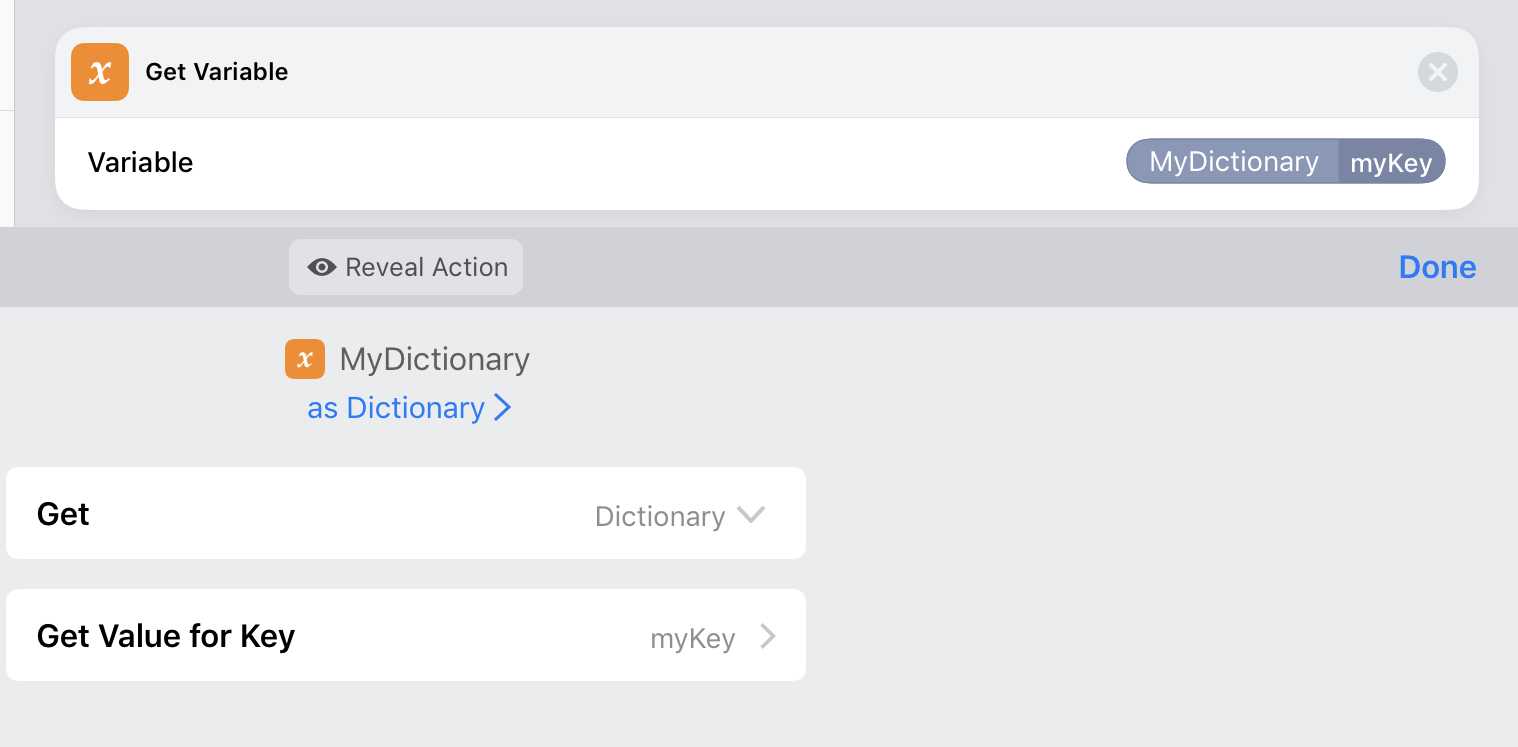
Actions Shown | GetVariable |
Setting Variables
->
can be used to set more than just magic variables, it can also automatically make SetVariable actions.
Text "My Text" -> v:MyNamedVariable
Image
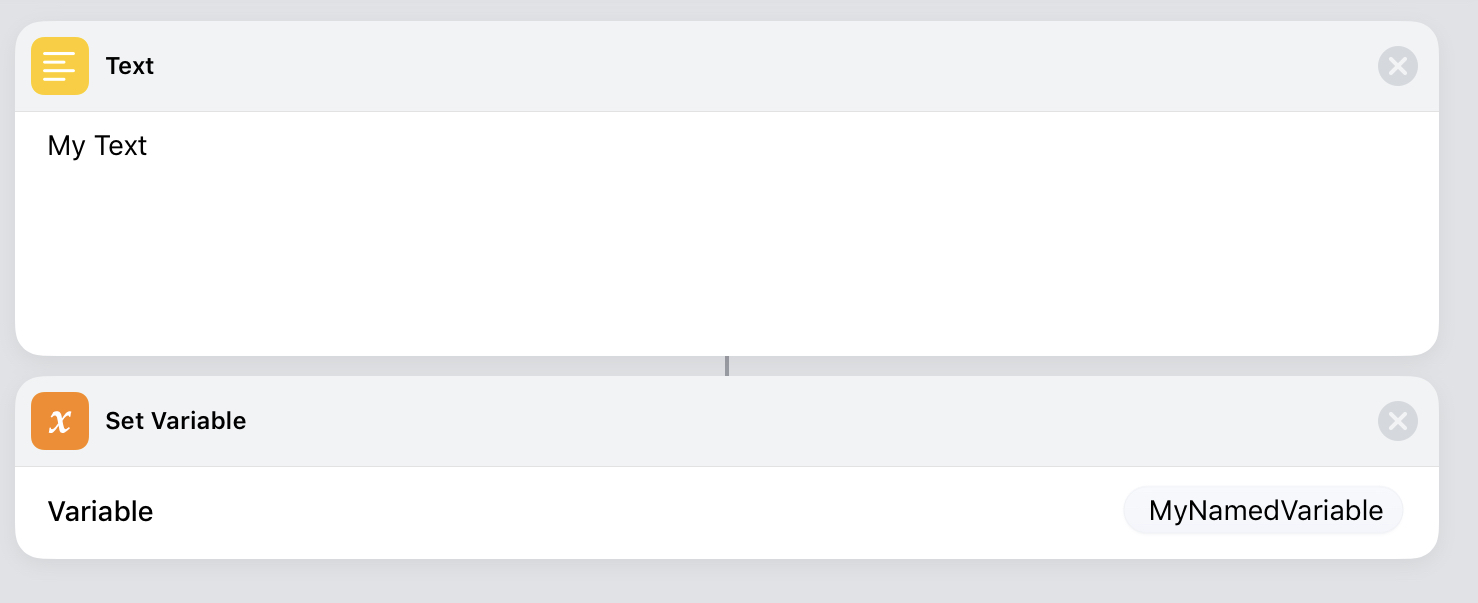
is equivalent to writing
Text "My Text"
SetVariable v:MyNamedVariable
Image
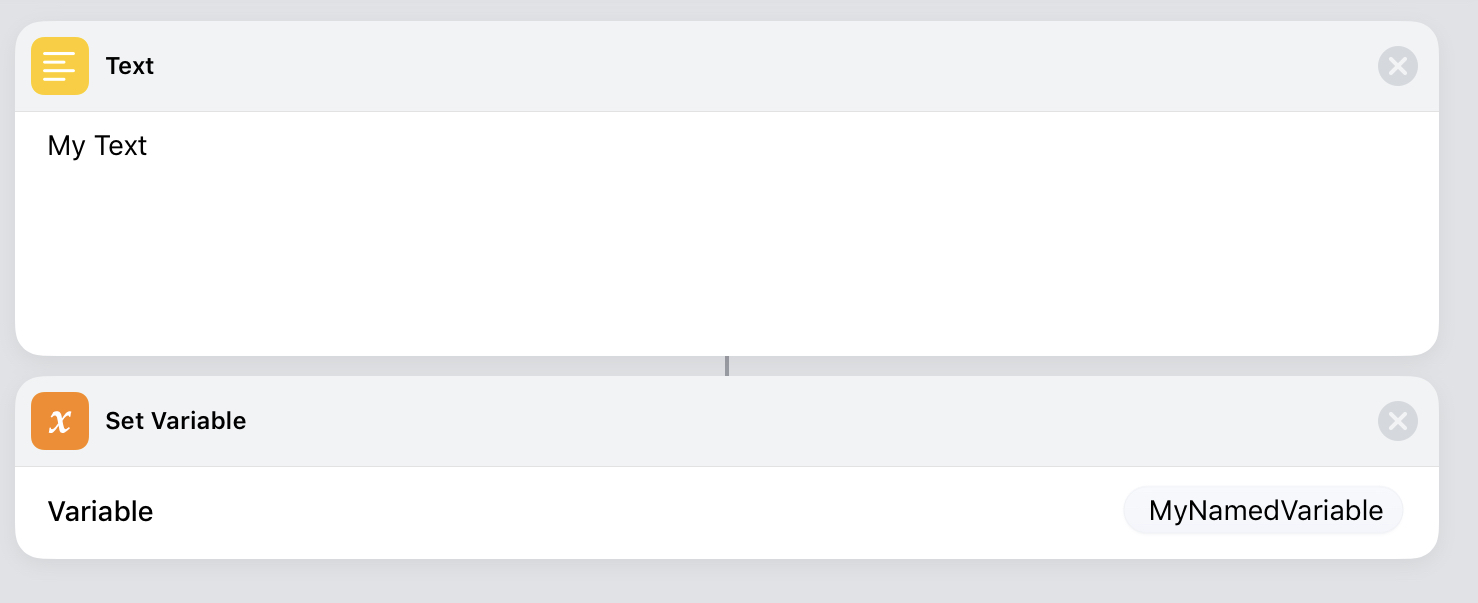
If you prefer, you can set variables before an action instead of after
mv:WowMagic = Text "My Text"
Image
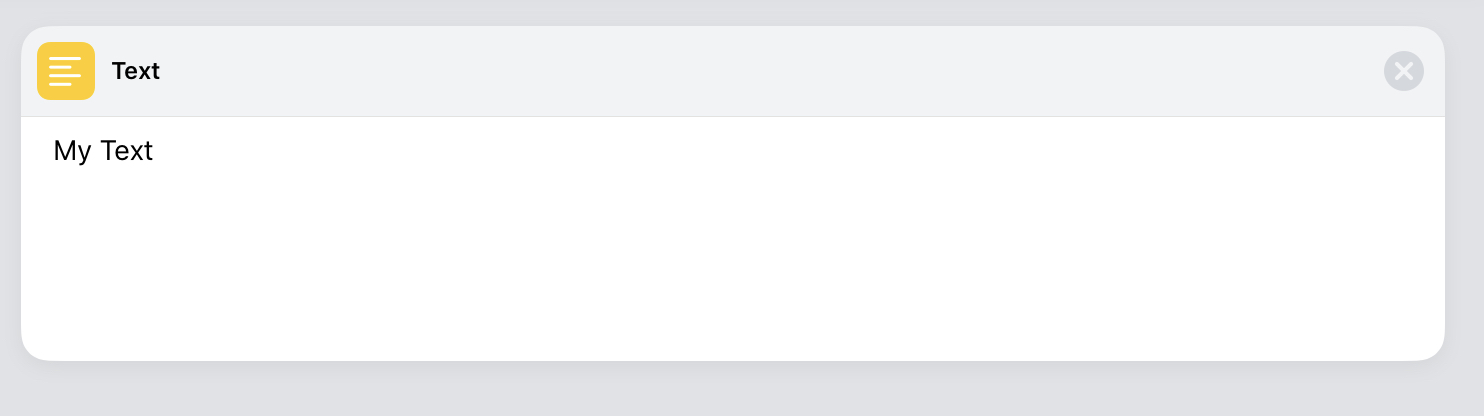
is exactly the same as using an arrow, except it’s in the format mv:variable = action
Text "My Text" -> mv:WowMagic
Image
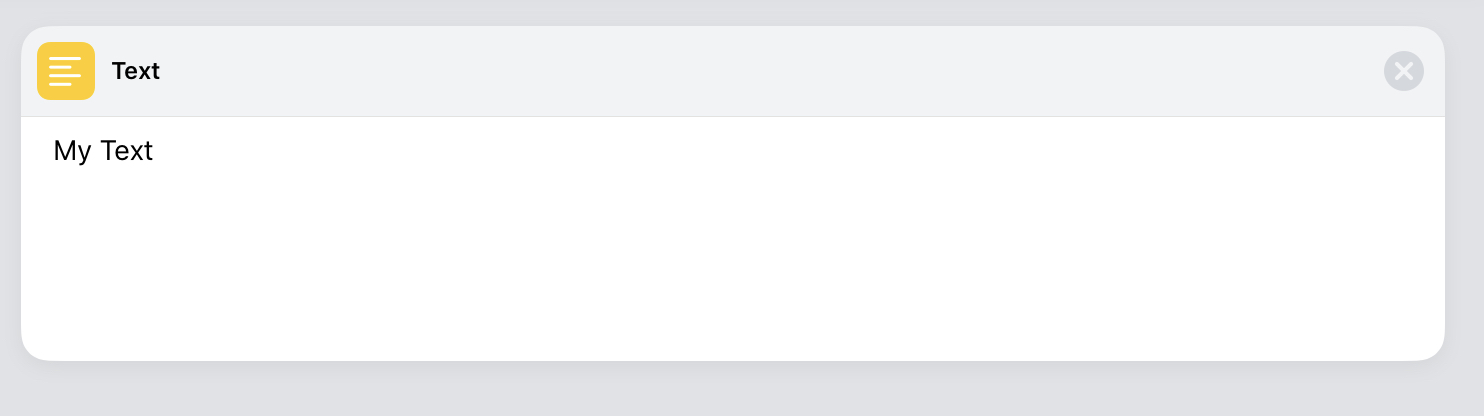
Actions Shown | Text | GetVariable |
ArgLists
Actions can usually only take up one line of text, unless you use a barlist. If you have many different arguments, it can be helpful to have actions span multiple lines. You can use an arglist for this.
getfile a{
service="iCloud Drive"
showdocumentpicker=false
filepath="/myfile.txt"
errorifnotfound=false
}
Image
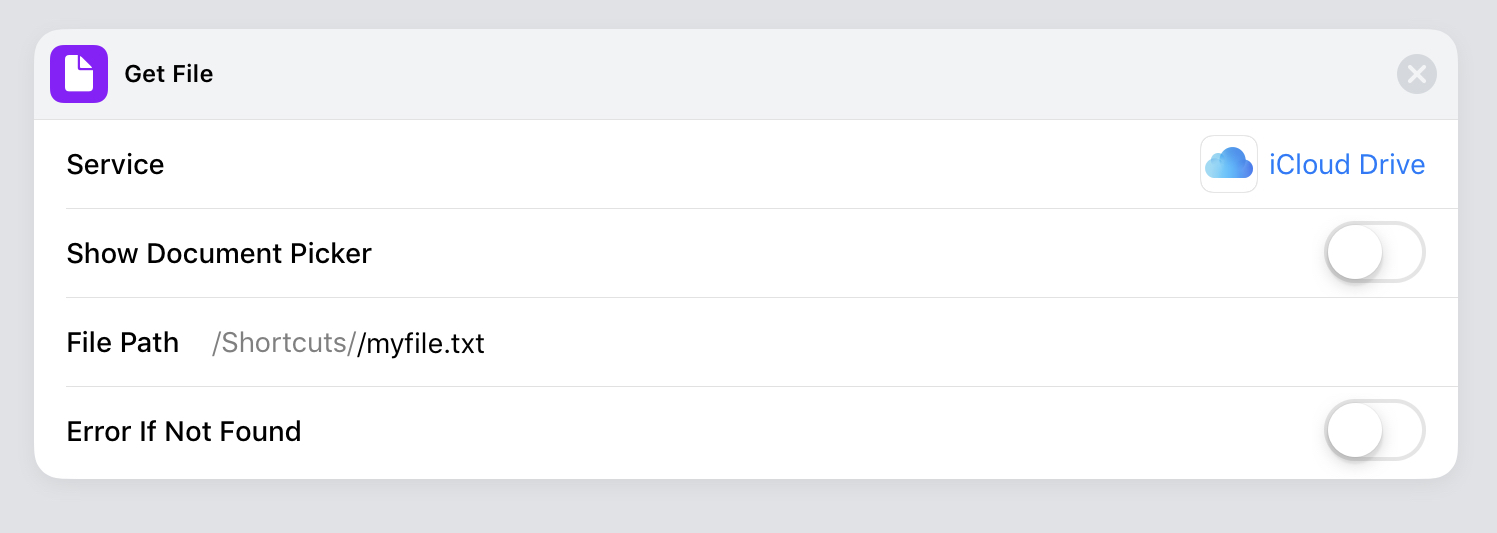
Actions shown | GetFile |
Special Variables
Shortcuts has 5 special variables, 2 of which seem to have no reason for existing.
- Ask When Run
s:AskWhenRun
(prompts the user to enter the field themselves) - Shortcut Input
s:ShortcutInput
(the input to the shortcut) - Action Input
s:ActionInput
(the input to the action) - Current Date
s:CurrentDate
(the current date) - Clipboard
s:Clipboard
(the contents of the clipboard)
Image
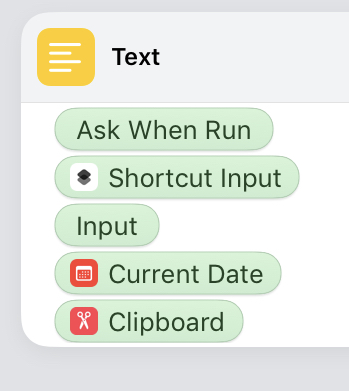
Macros and Parser Variables
The @set macro
Parser variables are variables that only exist in your scpl file. When the shortcut is generated, they are replaced with their value.
Set a parser variable with @set
, use a parser variable with @:variable
@set varname "value"
text "value is \(@:varname)"
Image

Parser variables inherit the properties of their values.
@set number 0.5
@set list [1, 2, 3]
number @:number
list @:list
Image

Parser variables can contain actions
@set myaction (text "hi")
@:myaction
Image

Parser variables can contain lists of actions
@set EscapeText @{
replacetext "\\" "\\\\"
replacetext (Text "\n") " "
replacetext ";" "\\;"
}
Text ";Hi there!\n\\"
@:EscapeText
Image

Parser variables can take input as a dictionary of parser variables to set
@set test (Text @:value)
@:test{value:"Hi!"}
Image

Use cases
In shortcuts, you can make functions by checking different inputs. To run these functions, normally it would take lots of code.
runShortcut v:ThisShortcut false ^(Dictionary{ action: Escape, text: "<the text>" })
You can define a macro/parser variable to insert that for you
setVariable v:ThisShortcut ^(text "Shortcut Name")
@set Escape (runShortcut v:ThisShortcut false ^(Dictionary{ action: Escape, text: @:text }))
AskForInput "Text to escape" -> mv:InputtedText
@:Escape{text: mv:InputtedText}
Image

The @foreach macro
Foreach will repeat an action multiple times
@foreach ["Item 1", "Item 2", "Item 3", "Item 4"] @{
Text @:repeatitem
}
Image

The @if
macro
The @if
macro allows you to do things if a boolean is true
@if true @{
text "True Condition"
} @{
text "False Condition"
}
The @elseif
macro
The @elseif
macro is a shorthand for chaining else ifs.
if Equals WifiOn
SetWifi true
@elseif Equals WifiOff
SetWifi false
@elseif Equals BluetoothOn
SetBluetooth true
else
SetBluetooth false
End
Image

The @error
macro
The @error
macro throws an error.
@error "There is a problem."
Image
Code does not compile, there is an errorThe @def
macro
The @def
macro allows you to define your own macros.
@def @printtext ["arg1", "arg2"] @{
ShowResult "Arg1 is \(@:arg1) and Arg2 is \(@:arg2)"
}
@PrintText arg1="Value1" arg2="Value2"
Image
